Python: Get the key, value and item in a dictionary
Python dictionary: Exercise-31 with Solution
Write a Python program to get the key, value and item in a dictionary.
Visual Presentation:
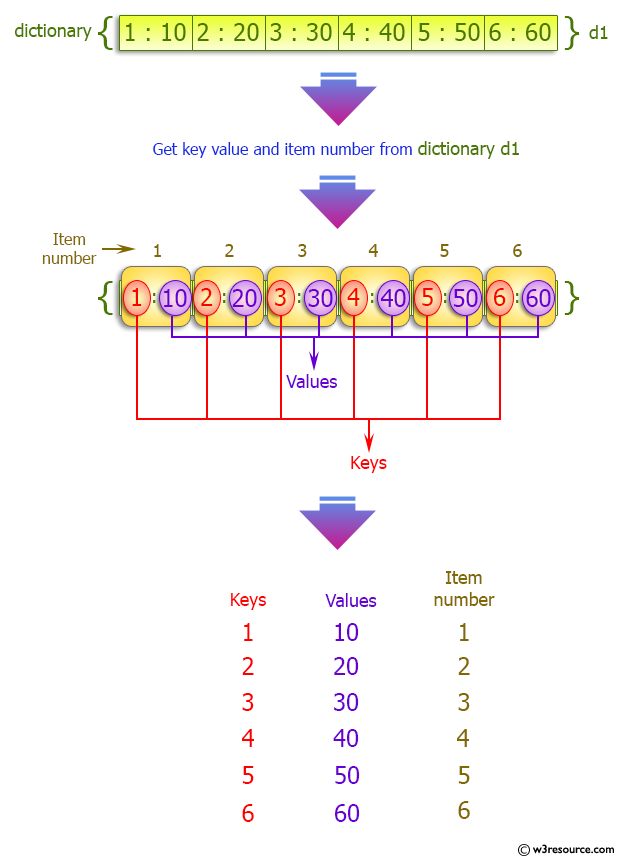
Sample Solution:
Python Code:
# Create a dictionary 'dict_num' with keys and values.
dict_num = {1: 10, 2: 20, 3: 30, 4: 40, 5: 50, 6: 60}
# Print a header for the output table.
print("key value count")
# Iterate through the key-value pairs in 'dict_num' using the 'enumerate' function.
# The 'enumerate' function assigns a count starting from 1 to each pair and unpacks them as (count, (key, value)).
for count, (key, value) in enumerate(dict_num.items(), 1):
# Print the key, value, and count in a formatted table.
print(key, ' ', value, ' ', count)
Sample Output:
key value count 1 10 1 2 20 2 3 30 3 4 40 4 5 50 5 6 60 6
Python Code Editor:
Previous: Write a Python program to get the top three items in a shop.
Next: Write a Python program to print a dictionary line by line.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/dictionary/python-data-type-dictionary-exercise-31.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics