Python: Replace dictionary values with their average
Write a Python program to replace dictionary values with their sums.
Visual Presentation:
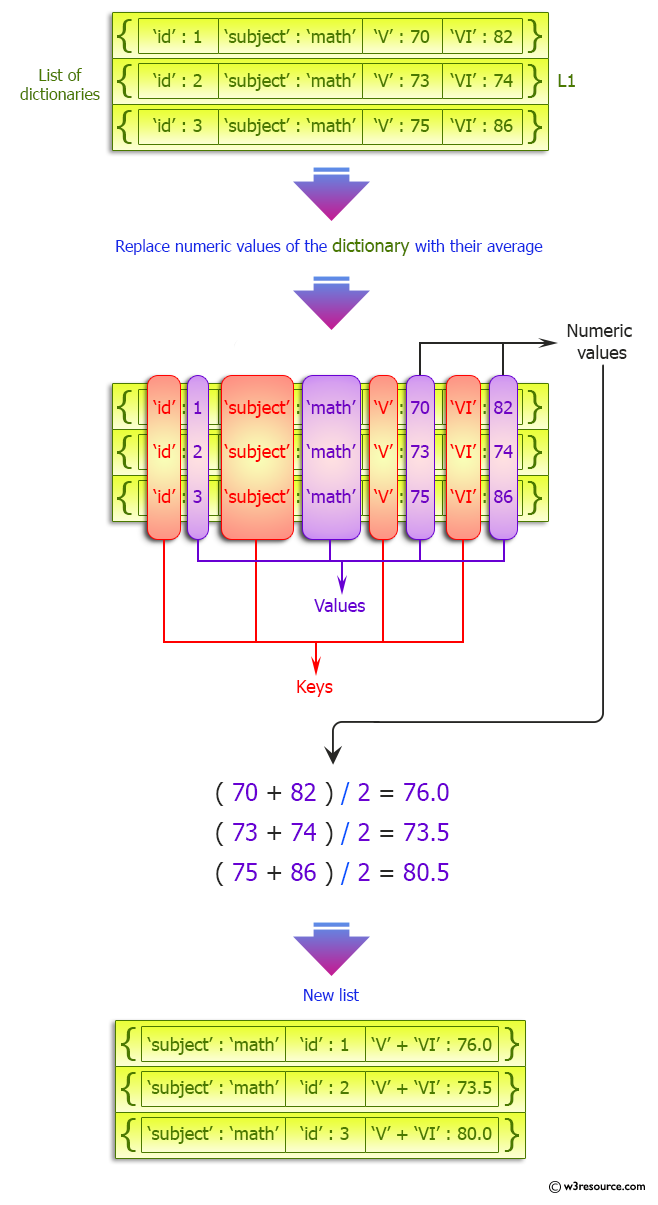
Sample Solution:
Python Code:
# Define a function 'sum_math_v_vi_average' that takes a list of dictionaries as input.
def sum_math_v_vi_average(list_of_dicts):
# Iterate through each dictionary in the input list.
for d in list_of_dicts:
# Extract and remove the 'V' and 'VI' values from the dictionary.
n1 = d.pop('V')
n2 = d.pop('VI')
# Calculate the average of 'V' and 'VI' and store it as 'V+VI' in the dictionary.
d['V+VI'] = (n1 + n2) / 2
# Return the modified list of dictionaries.
return list_of_dicts
# Create a list of dictionaries 'student_details' containing student information.
student_details = [
{'id': 1, 'subject': 'math', 'V': 70, 'VI': 82},
{'id': 2, 'subject': 'math', 'V': 73, 'VI': 74},
{'id': 3, 'subject': 'math', 'V': 75, 'VI': 86}
]
# Call the 'sum_math_v_vi_average' function and print the modified list of student details.
print(sum_math_v_vi_average(student_details))
Sample Output:
[{'subject': 'math', 'id': 1, 'V+VI': 76.0}, {'subject': 'math', 'id': 2, 'V+VI': 73.5}, {'subject': 'math', ' id': 3, 'V+VI': 80.5}]
Python Code Editor:
Previous: Write a Python program to create a dictionary from two lists without losing duplicate values.
Next: Write a Python program to match key values in two dictionaries.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics