Python: Match key values in two dictionaries
Python dictionary: Exercise-38 with Solution
Write a Python program to match key values in two dictionaries.
Visual Presentation:
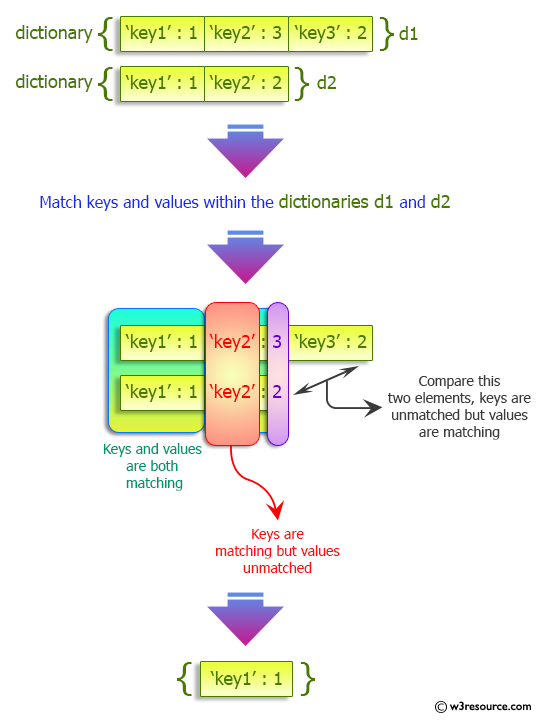
Sample Solution:
Python Code:
# Create two dictionaries 'x' and 'y' with key-value pairs.
x = {'key1': 1, 'key2': 3, 'key3': 2}
y = {'key1': 1, 'key2': 2}
# Use set operations to find the common key-value pairs between 'x' and 'y'.
# Iterate through the common key-value pairs using a for loop.
for (key, value) in set(x.items()) & set(y.items()):
# Print a message indicating that the key and value are present in both 'x' and 'y'.
print('%s: %s is present in both x and y' % (key, value))
Sample Output:
key1: 1 is present in both x and y
Python Code Editor:
Previous: Write a Python program to replace dictionary values with their sum.
Next: Write a Python program to store a given dictionary in a json file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/dictionary/python-data-type-dictionary-exercise-38.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics