Python: Create a dictionary of keys x, y, and z
Python dictionary: Exercise-40 with Solution
Write a Python program to create a dictionary of keys x, y, and z where each key has as value a list from 11-20, 21-30, and 31-40 respectively. Access the fifth value of each key from the dictionary.
Visual Presentation:
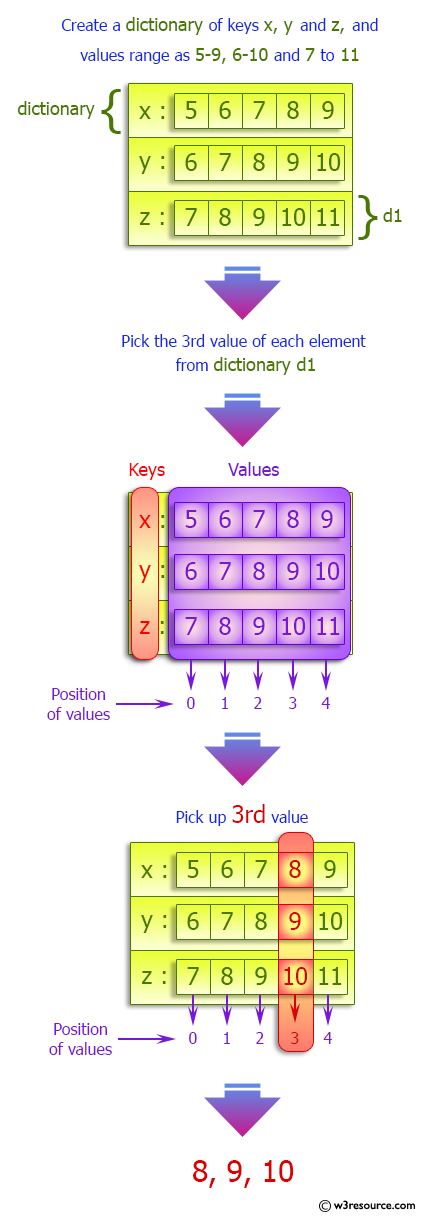
Sample Solution:
Python Code:
# Import the 'pprint' function from the 'pprint' module to pretty-print dictionaries.
from pprint import pprint
# Create a dictionary 'dict_nums' with keys 'x', 'y', and 'z', and lists of numbers as values.
dict_nums = dict(x=list(range(11, 20)), y=list(range(21, 30)), z=list(range(31, 40)))
# Pretty-print the 'dict_nums' dictionary to improve readability.
pprint(dict_nums)
# Print the value at the 4th index of the 'x', 'y', and 'z' lists in 'dict_nums'.
print(dict_nums["x"][4])
print(dict_nums["y"][4])
print(dict_nums["z"][4])
# Iterate through the key-value pairs in 'dict_nums' and print the key and its associated value.
for k, v in dict_nums.items():
print(k, "has value", v)
Sample Output:
{'x': [11, 12, 13, 14, 15, 16, 17, 18, 19], 'y': [21, 22, 23, 24, 25, 26, 27, 28, 29], 'z': [31, 32, 33, 34, 35, 36, 37, 38, 39]} 15 25 35 x has value [11, 12, 13, 14, 15, 16, 17, 18, 19] y has value [21, 22, 23, 24, 25, 26, 27, 28, 29] z has value [31, 32, 33, 34, 35, 36, 37, 38, 39]
Flowchart:
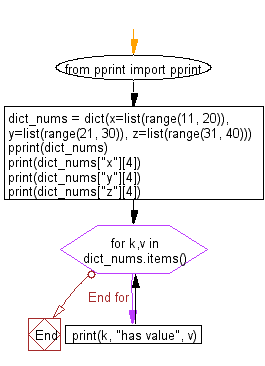
Python Code Editor:
Previous: Write a Python program to store a given dictionary in a json file.
Next: Write a Python program to drop empty Items from a given Dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/dictionary/python-data-type-dictionary-exercise-40.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics