Python: Convert more than one list to nested dictionary
43. Convert Multiple Lists to a Nested Dictionary
Write a Python program to convert more than one list to a nested dictionary.
Visual Presentation:
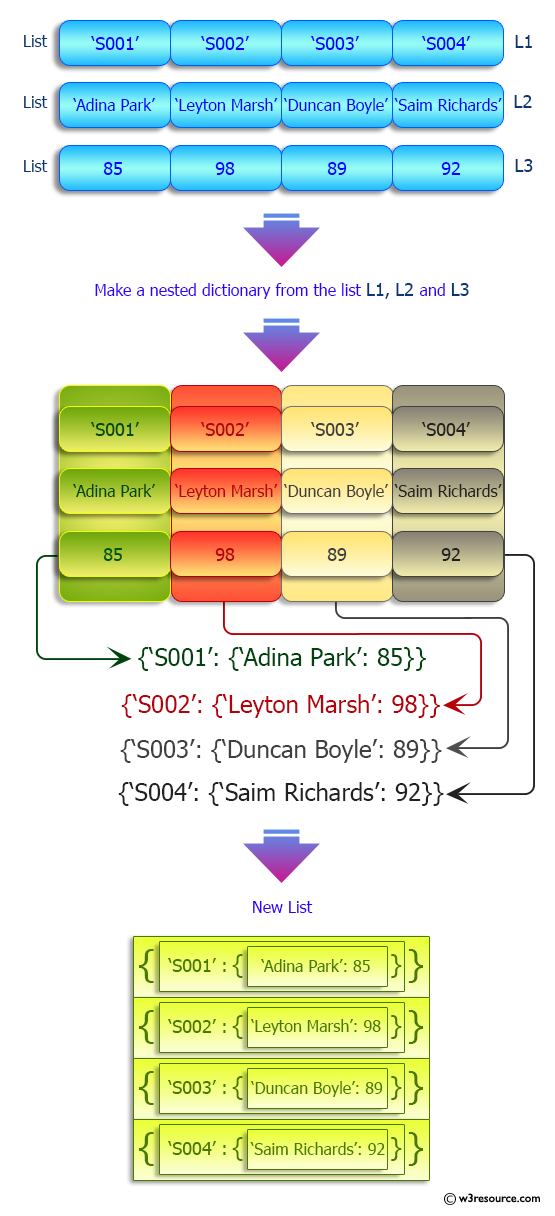
Sample Solution:
Python Code:
Sample Output:
Original strings: ['S001', 'S002', 'S003', 'S004'] ['Adina Park', 'Leyton Marsh', 'Duncan Boyle', 'Saim Richards'] [85, 98, 89, 92] Nested dictionary: [{'S001': {'Adina Park': 85}}, {'S002': {'Leyton Marsh': 98}}, {'S003': {'Duncan Boyle': 89}}, {'S004': {'Saim Richards': 92}}]
Flowchart:
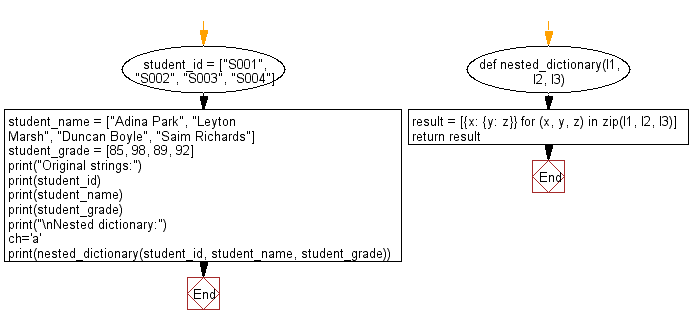
For more Practice: Solve these Related Problems:
- Write a Python program to merge three lists into a list of nested dictionaries using zip() and list comprehension.
- Write a Python function that takes three parallel lists (IDs, names, scores) and returns a nested dictionary for each item.
- Write a Python program to convert multiple lists into a nested dictionary where each outer key maps to a dictionary of inner key-value pairs.
- Write a Python program to build a nested dictionary from several lists while ensuring proper pairing of elements.
Go to:
Previous: Write a Python program to filter a dictionary based on values.
Next: Write a Python program to filter the height and width of students, which are stored in a dictionary.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.