Python: Filter the height and width of students which are stored in a dictionary
Write a Python program to filter the height and width of students, which are stored in a dictionary.
Visualal Presentation:
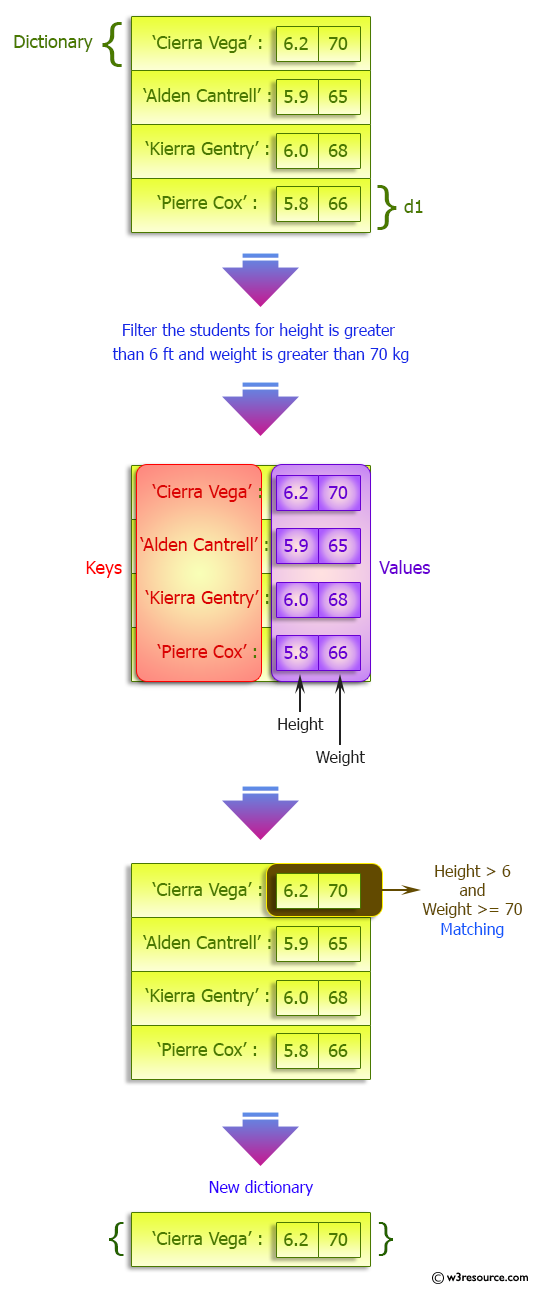
Sample Solution:
Python Code:
# Define a function 'filter_data' that filters students based on height and weight criteria.
def filter_data(students):
# Use a dictionary comprehension to create a new dictionary 'result'.
# Include key-value pairs from 'students' where the height (s[0]) is greater than or equal to 6.0
# and the weight (s[1]) is greater than or equal to 70.
result = {k: s for k, s in students.items() if s[0] >= 6.0 and s[1] >= 70}
return result
# Create a dictionary 'students' with student names as keys and tuples of height and weight as values.
students = {
'Cierra Vega': (6.2, 70),
'Alden Cantrell': (5.9, 65),
'Kierra Gentry': (6.0, 68),
'Pierre Cox': (5.8, 66)
}
# Print a message indicating the start of the code section and the original dictionary.
print("Original Dictionary:")
print(students)
# Print a message indicating the intention to filter students based on height and weight criteria.
print("\nHeight > 6ft and Weight > 70kg:")
# Call the 'filter_data' function to filter students based on the specified criteria.
# Print the resulting filtered dictionary.
print(filter_data(students))
Sample Output:
Original Dictionary: {'Cierra Vega': (6.2, 70), 'Alden Cantrell': (5.9, 65), 'Kierra Gentry': (6.0, 68), 'Pierre Cox': (5.8, 66)} Height > 6ft and Weight> 70kg: {'Cierra Vega': (6.2, 70)}
Flowchart:
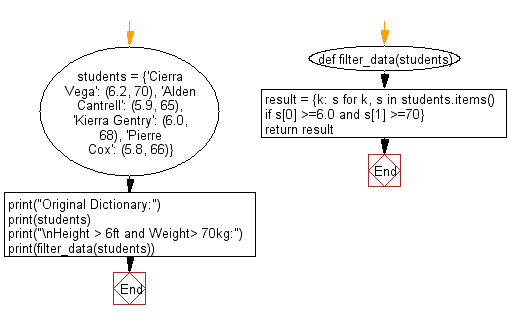
Python Code Editor:
Previous: Write a Python program to convert more than one list to nested dictionary.
Next: Write a Python program to check all values are same in a dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics