Python: Grouping a sequence of key-value pairs into a dictionary of lists
Python dictionary: Exercise-46 with Solution
Write a Python program to create a dictionary grouping a sequence of key-value pairs into a dictionary of lists.
Visual Presentation:
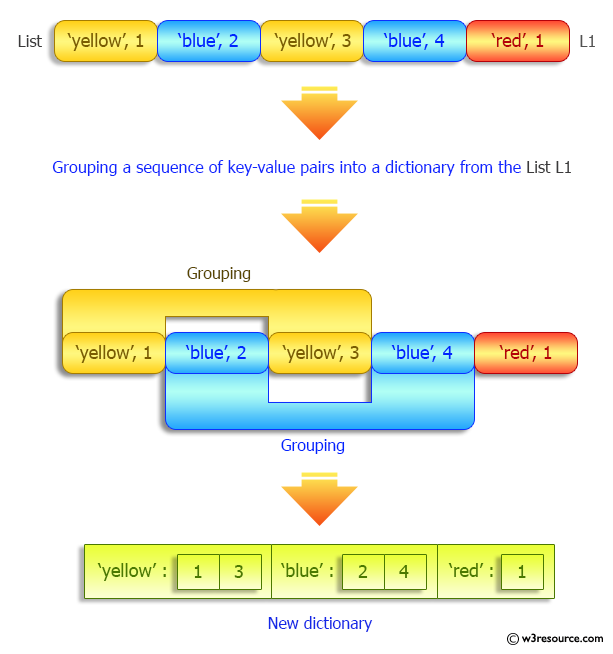
Sample Solution:
Python Code:
# Define a function 'grouping_dictionary' that groups a sequence of key-value pairs into a dictionary of lists.
def grouping_dictionary(l):
# Create an empty dictionary 'result' to store the grouped data.
result = {}
for k, v in l:
# Use 'setdefault' to append values 'v' to the list associated with key 'k'.
result.setdefault(k, []).append(v)
return result
# Create a list 'colors' containing key-value pairs (color, value).
colors = [('yellow', 1), ('blue', 2), ('yellow', 3), ('blue', 4), ('red', 1)]
# Print a message indicating the start of the code section and the original list.
print("Original list:")
print(colors)
# Print a message indicating the intention to group the key-value pairs into a dictionary of lists.
print("\nGrouping a sequence of key-value pairs into a dictionary of lists:")
# Call the 'grouping_dictionary' function to group the key-value pairs and print the resulting dictionary.
print(grouping_dictionary(colors))
Sample Output:
Original list: [('yellow', 1), ('blue', 2), ('yellow', 3), ('blue', 4), ('red', 1)] Grouping a sequence of key-value pairs into a dictionary of lists: {'yellow': [1, 3], 'blue': [2, 4], 'red': [1]}
Flowchart:
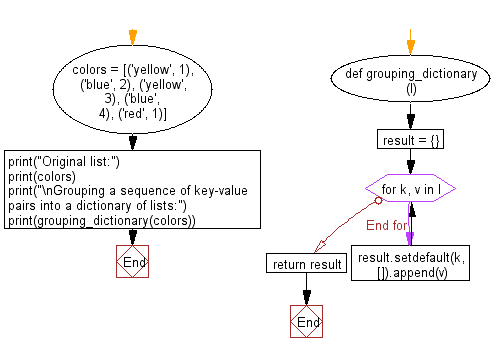
Python Code Editor:
Previous: Write a Python program to check all values are same in a dictionary.
Next: Write a Python program to split a given dictionary of lists into list of dictionaries.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/dictionary/python-data-type-dictionary-exercise-46.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics