Python: Split a given dictionary of lists into list of dictionaries
Python dictionary: Exercise-47 with Solution
Write a Python program to split a given dictionary of lists into lists of dictionaries.
Visual Presentation:
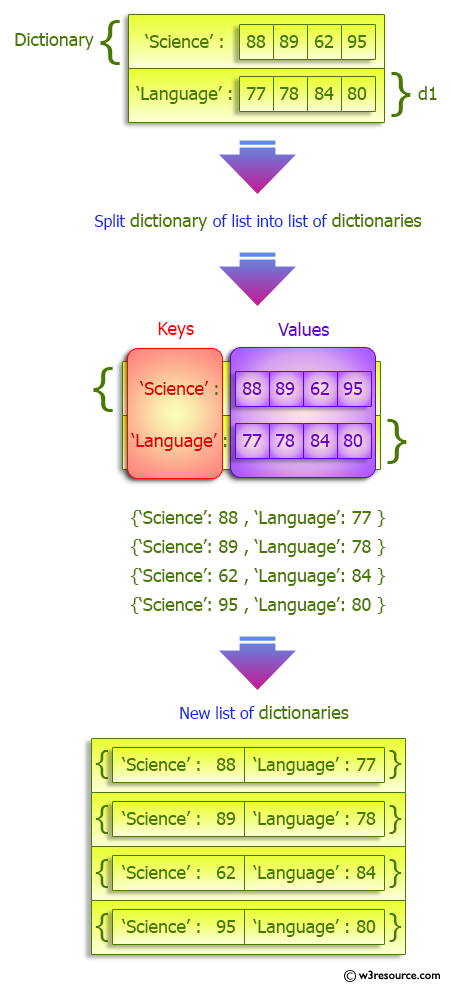
Sample Solution:
Python Code:
# Define a function 'list_of_dicts' that splits a dictionary of lists into a list of dictionaries.
def list_of_dicts(marks):
# Get the keys (subjects) from the 'marks' dictionary.
keys = marks.keys()
# Use the 'zip' function to transpose the lists of marks into tuples.
vals = zip(*[marks[k] for k in keys])
# Create a list of dictionaries by zipping the keys and the transposed tuples.
result = [dict(zip(keys, v)) for v in vals]
return result
# Create a dictionary 'marks' with subjects as keys and lists of marks as values.
marks = {'Science': [88, 89, 62, 95], 'Language': [77, 78, 84, 80]}
# Print a message indicating the start of the code section and the original dictionary of lists.
print("Original dictionary of lists:")
print(marks)
# Print a message indicating the intention to split the dictionary of lists into a list of dictionaries.
print("\nSplit said dictionary of lists into a list of dictionaries:")
# Call the 'list_of_dicts' function to split the dictionary of lists and print the resulting list of dictionaries.
print(list_of_dicts(marks))
Sample Output:
Original dictionary of lists: {'Science': [88, 89, 62, 95], 'Language': [77, 78, 84, 80]} Split said dictionary of lists into list of dictionaries: [{'Science': 88, 'Language': 77}, {'Science': 89, 'Language': 78}, {'Science': 62, 'Language': 84}, {'Science': 95, 'Language': 80}]
Flowchart:
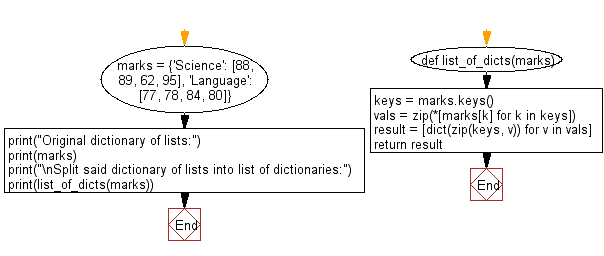
Python Code Editor:
Previous: Write a Python program to create a dictionary grouping a sequence of key-value pairs into a dictionary of lists.
Next: Write a Python program to remove a specified dictionary from a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/dictionary/python-data-type-dictionary-exercise-47.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics