Python: Update the list values within a given dictionary
Python dictionary: Exercise-51 with Solution
A Python Dictionary contains List as a value. Write a Python program to update the list values in the said dictionary.
Visual Presentation:
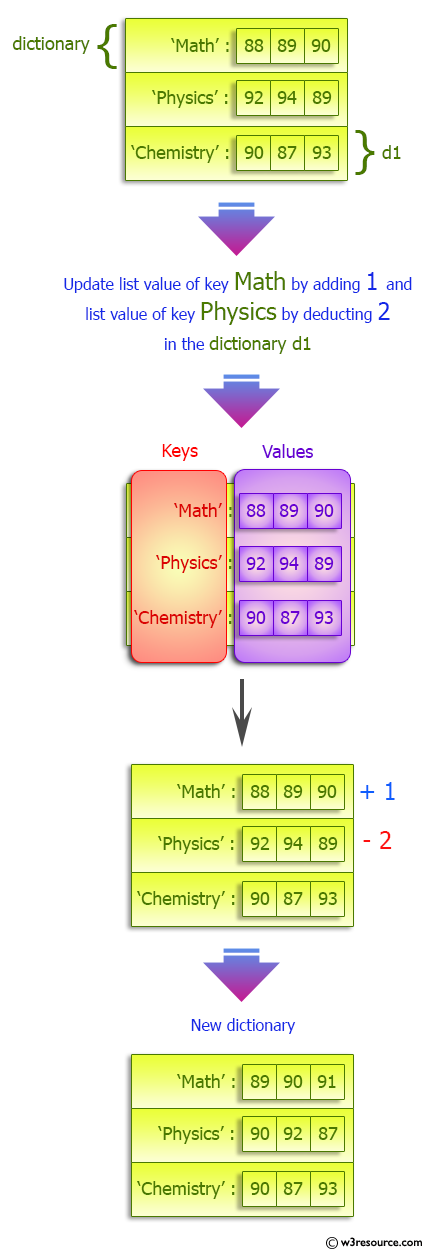
Sample Solution:
Python Code:
# Define a function 'test' that updates the list values within a dictionary.
def test(dictionary):
# Update the 'Math' key's list values by adding 1 to each element using a list comprehension.
dictionary['Math'] = [x + 1 for x in dictionary['Math']]
# Update the 'Physics' key's list values by subtracting 2 from each element using a list comprehension.
dictionary['Physics'] = [x - 2 for x in dictionary['Physics']]
return dictionary
# Create a dictionary 'dictionary' with keys 'Math', 'Physics', and 'Chemistry', and lists as values.
dictionary = {
'Math': [88, 89, 90],
'Physics': [92, 94, 89],
'Chemistry': [90, 87, 93]
}
# Print a message indicating the start of the code section and the original dictionary.
print("\nOriginal Dictionary:")
print(dictionary)
# Print a message indicating the intention to update the list values in the dictionary.
print("\nUpdate the list values of the said dictionary:")
# Call the 'test' function to update the list values within the dictionary and print the resulting dictionary.
print(test(dictionary))
Sample Output:
Original Dictionary: {'Math': [88, 89, 90], 'Physics': [92, 94, 89], 'Chemistry': [90, 87, 93]} Update the list values of the said dictionary: {'Math': [89, 90, 91], 'Physics': [90, 92, 87], 'Chemistry': [90, 87, 93]}
Flowchart:
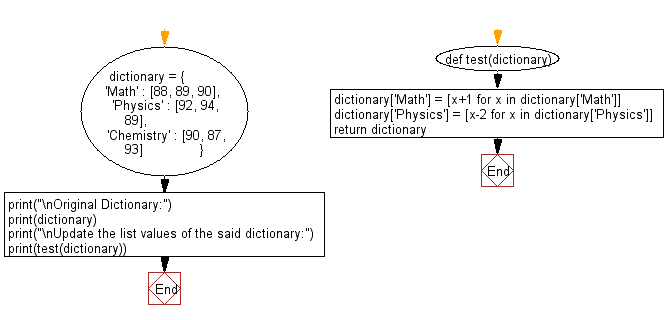
Python Code Editor:
Previous: A Python Dictionary contains List as value. Write a Python program to clear the list values in the said dictionary.
Next: Write a Python program to extract a list of values from a given list of dictionaries.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/dictionary/python-data-type-dictionary-exercise-51.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics