Python: Filter even numbers from a given dictionary values
Write a Python program to filter even numbers from a dictionary of values.
Visual Presentation:
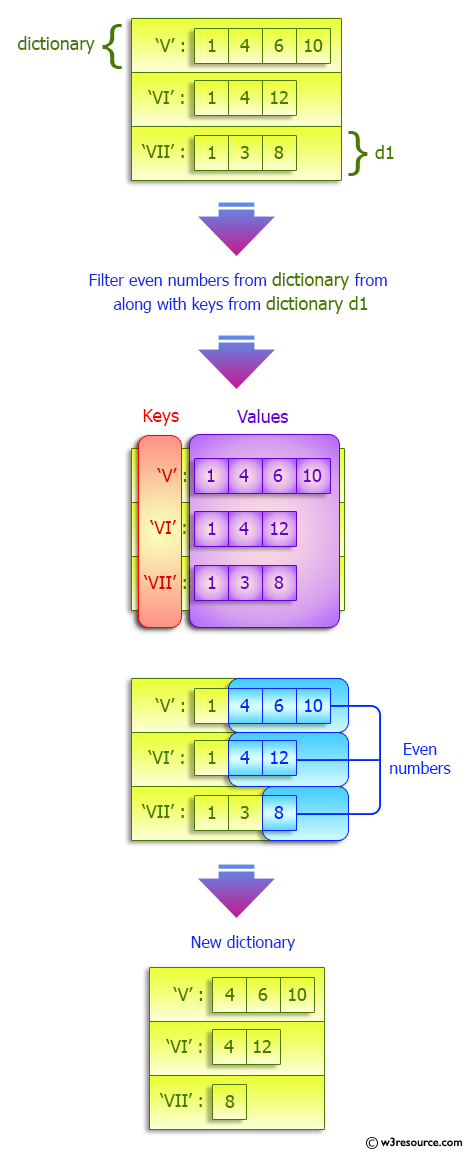
Sample Solution:
Python Code:
# Define a function 'test' that filters even numbers from dictionary values.
def test(dictt):
# Use a dictionary comprehension to filter even numbers for each value in the dictionary.
result = {key: [idx for idx in val if not idx % 2] for key, val in dictt.items()}
return result
# Create a dictionary 'students' with string keys (class names) and lists of integers as values.
students = {'V' : [1, 4, 6, 10], 'VI' : [1, 4, 12], 'VII' : [1, 3, 8]}
# Print a message indicating the start of the code section and the dictionary being processed.
print("\nOriginal Dictionary:")
print(students)
# Call the 'test' function to filter even numbers from dictionary values and print the result.
print("Filter even numbers from said dictionary values:")
print(test(students))
# Create another dictionary 'students' with string keys (class names) and lists of integers as values.
students = {'V' : [1, 3, 5], 'VI' : [1, 5], 'VII' : [2, 7, 9]}
# Print a message indicating the start of the code section and the new dictionary being processed.
print("\nOriginal Dictionary:")
print(students)
# Call the 'test' function to filter even numbers from dictionary values and print the result.
print("Filter even numbers from said dictionary values:")
print(test(students))
Sample Output:
Original Dictionary: {'V': [1, 4, 6, 10], 'VI': [1, 4, 12], 'VII': [1, 3, 8]} Filter even numbers from said dictionary values: {'V': [4, 6, 10], 'VI': [4, 12], 'VII': [8]} Original Dictionary: {'V': [1, 3, 5], 'VI': [1, 5], 'VII': [2, 7, 9]} Filter even numbers from said dictionary values: {'V': [], 'VI': [], 'VII': [2]}
Flowchart:
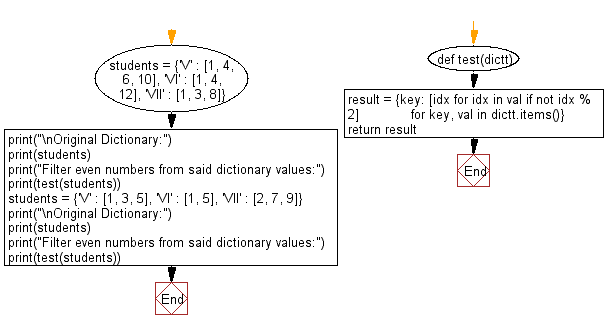
Python Code Editor:
Previous: Write a Python program to convert a given dictionary into a list of lists.
Next: Write a Python program to get all combinations of key-value pairs in a given dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics