Python: Combinations of key-value pairs in a given dictionary
58. Get All Combinations of Key-Value Pairs in a Dictionary
Write a Python program to get all combinations of key-value pairs in a given dictionary.
Visual Presentation:
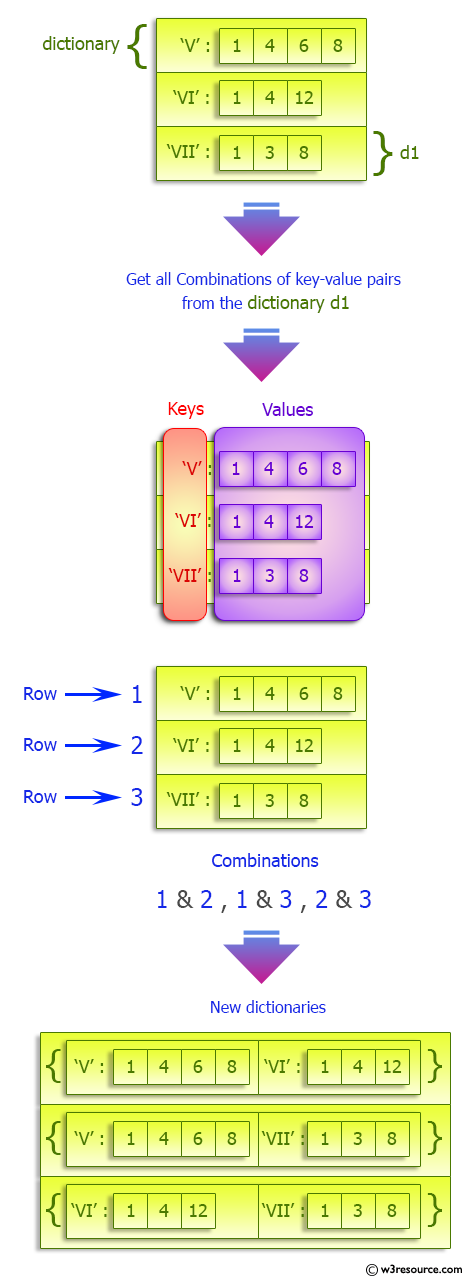
Sample Solution:
Python Code:
# Import the 'itertools' module to use its combination functions.
import itertools
# Define a function 'test' that takes a dictionary 'dictt' as an argument.
def test(dictt):
# Generate all combinations of key-value pairs in the dictionary.
# Convert each combination into a dictionary and return a list of dictionaries.
result = list(map(dict, itertools.combinations(dictt.items(), 2)))
return result
# Create a dictionary 'students' where the keys are grades ('V', 'VI', 'VII') and the values are lists of integers.
students = {'V': [1, 4, 6, 10], 'VI': [1, 4, 12], 'VII': [1, 3, 8]}
# Print a message indicating the start of the code section and the original dictionary.
print("\nOriginal Dictionary:")
print(students)
# Print a message indicating the purpose and generate all combinations of key-value pairs for the 'students' dictionary using the 'test' function.
print("\nCombinations of key-value pairs of the said dictionary:")
print(test(students))
# Create another dictionary 'students' with different values.
students = {'V': [1, 3, 5], 'VI': [1, 5]}
# Print a message indicating the start of the code section and the original dictionary.
print("\nOriginal Dictionary:")
print(students)
# Print a message indicating the purpose and generate all combinations of key-value pairs for the new 'students' dictionary using the 'test' function.
print("\nCombinations of key-value pairs of the said dictionary:")
print(test(students))
Sample Output:
Original Dictionary: {'V': [1, 4, 6, 10], 'VI': [1, 4, 12], 'VII': [1, 3, 8]} Combinations of key-value pairs of the said dictionary: [{'V': [1, 4, 6, 10], 'VI': [1, 4, 12]}, {'V': [1, 4, 6, 10], 'VII': [1, 3, 8]}, {'VI': [1, 4, 12], 'VII': [1, 3, 8]}] Original Dictionary: {'V': [1, 3, 5], 'VI': [1, 5]} Combinations of key-value pairs of the said dictionary: [{'V': [1, 3, 5], 'VI': [1, 5]}]
Flowchart:
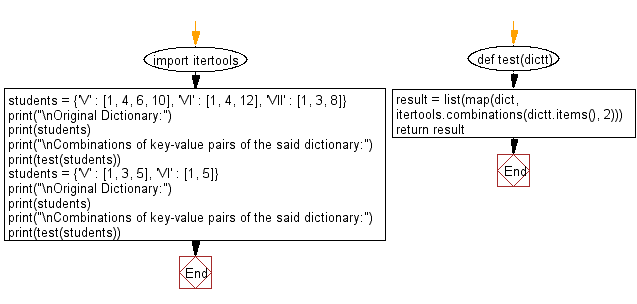
For more Practice: Solve these Related Problems:
- Write a Python program to generate all possible combinations of two keys from a dictionary using itertools.combinations.
- Write a Python program to create a list of dictionaries, each containing a unique combination of key-value pairs of a given size.
- Write a Python program to implement a function that returns all subsets of key-value pairs from a dictionary.
- Write a Python program to use recursion to generate combinations of keys and return corresponding sub-dictionaries.
Go to:
Previous: Write a Python program to filter even numbers from a given dictionary values.
Next: Write a Python program to find the specified number of maximum values in a given dictionary.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.