Python: Find the specified number of maximum values in a given dictionary
Python dictionary: Exercise-59 with Solution
Write a Python program to find the specified number of maximum values in a given dictionary.
Visual Presentation:
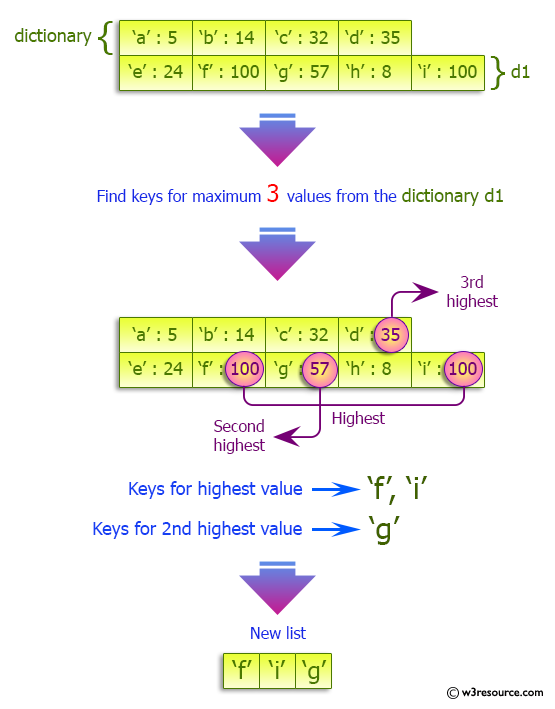
Sample Solution:
Python Code:
def test(dictt, N):
result = sorted(dictt, key=dictt.get, reverse=True)[:N]
return result
dictt = {'a':5, 'b':14, 'c': 32, 'd':35, 'e':24, 'f': 100, 'g':57, 'h':8, 'i': 100}
print("\nOriginal Dictionary:")
print(dictt)
N = 1
print("\n",N,"maximum value(s) in the said dictionary:")
print(test(dictt, N))
N = 2
print("\n",N,"maximum value(s) in the said dictionary:")
print(test(dictt, N))
N = 5
print("\n",N,"maximum value(s) in the said dictionary:")
print(test(dictt, N))
Sample Output:
Original Dictionary: {'a': 5, 'b': 14, 'c': 32, 'd': 35, 'e': 24, 'f': 100, 'g': 57, 'h': 8, 'i': 100} 1 maximum value(s) in the said dictionary: ['f'] 2 maximum value(s) in the said dictionary: ['f', 'i'] 5 maximum value(s) in the said dictionary: ['f', 'i', 'g', 'd', 'c']
Flowchart:
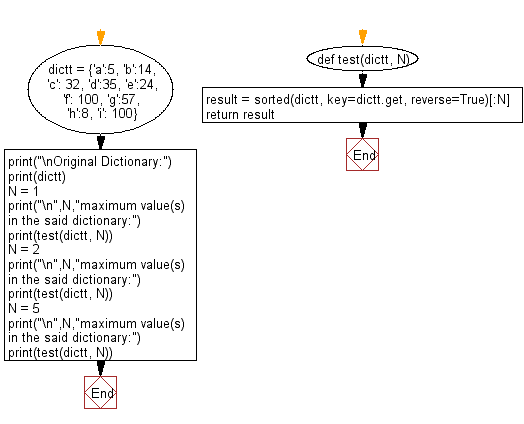
Python Code Editor:
Previous: Write a Python program to get all combinations of key-value pairs in a given dictionary.
Next: Write a Python program to find shortest list of values with the keys in a given dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/dictionary/python-data-type-dictionary-exercise-59.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics