Python: Find shortest list of values with the keys of a dictionary
Python dictionary: Exercise-60 with Solution
Write a Python program to find the shortest list of values for the keys in a given dictionary.
Visual Presentation:
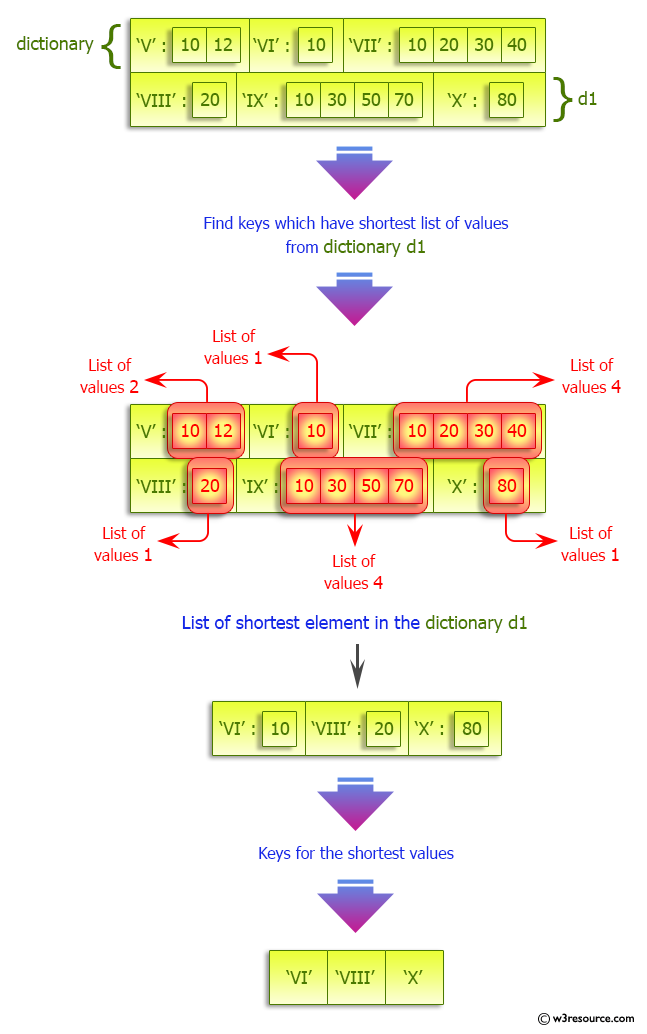
Sample Solution:
Python Code:
# Define a function 'test' that takes a dictionary 'dictt' as an argument.
def test(dictt):
# Define a minimum value for the length of sublists (1 in this case).
min_value = 1
# Create a list of keys 'k' for which the length of the associated list 'v' is equal to 'min_value'.
result = [k for k, v in dictt.items() if len(v) == min_value]
# Return the list of keys.
return result
# Create a dictionary 'dictt' where the keys represent grades ('V', 'VI', etc.) and the values are lists of integers.
dictt = {
'V': [10, 12],
'VI': [10],
'VII': [10, 20, 30, 40],
'VIII': [20],
'IX': [10, 30, 50, 70],
'X': [80]
}
# Print a message indicating the start of the code section and display the original dictionary.
print("\nOriginal Dictionary:")
print(dictt)
# Print a message indicating the purpose and generate a list of keys associated with the shortest lists in the 'dictt' dictionary using the 'test' function.
print("\nShortest list of values with the keys of the said dictionary:")
print(test(dictt))
Sample Output:
Original Dictionary: {'V': [10, 12], 'VI': [10], 'VII': [10, 20, 30, 40], 'VIII': [20], 'IX': [10, 30, 50, 70], 'X': [80]} Shortest list of values with the keys of the said dictionary: ['VI', 'VIII', 'X']
Flowchart:
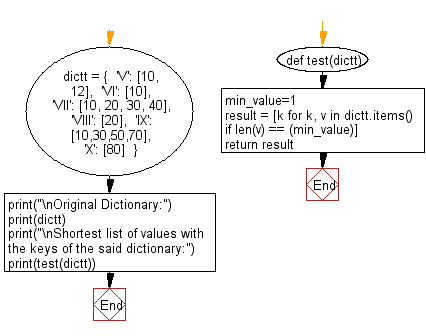
Python Code Editor:
Previous: Write a Python program to find the specified number of maximum values in a given dictionary.
Next: Write a Python program to count the frequency in a given dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/dictionary/python-data-type-dictionary-exercise-60.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics