Python: Count the frequency in a given dictionary
61. Count Frequency of a Dictionary's Values
Write a Python program to count the frequency of a dictionary.
Visual Presentation:
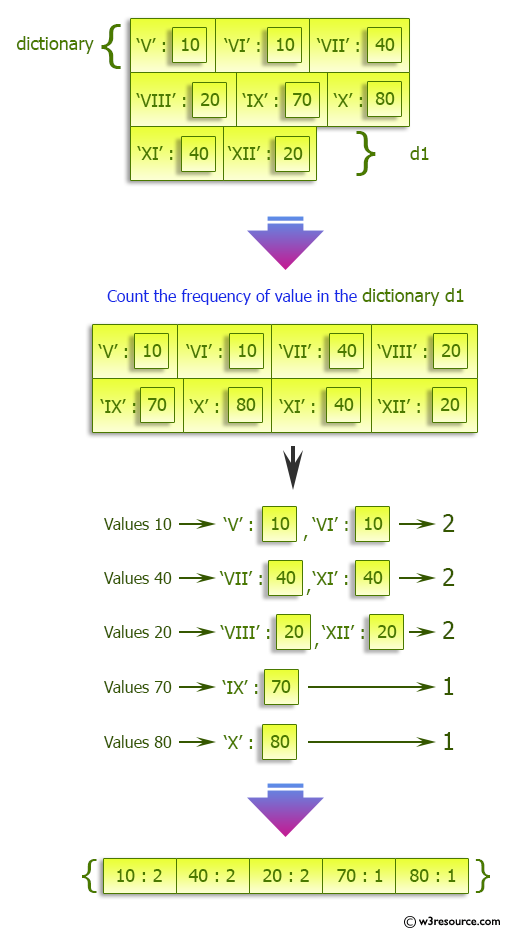
Sample Solution:
Python Code:
# Import the 'Counter' class from the 'collections' module.
from collections import Counter
# Define a function 'test' that takes a dictionary 'dictt' as an argument.
def test(dictt):
# Use the 'Counter' class to count the frequency of values in the dictionary.
result = Counter(dictt.values())
# Return the result as a Counter object.
return result
# Create a dictionary 'dictt' where the keys represent grades ('V', 'VI', etc.) and the values are integers.
dictt = {
'V': 10,
'VI': 10,
'VII': 40,
'VIII': 20,
'IX': 70,
'X': 80,
'XI': 40,
'XII': 20
}
# Print a message indicating the start of the code section and display the original dictionary.
print("\nOriginal Dictionary:")
print(dictt)
# Print a message indicating the purpose and generate a frequency count of values in the 'dictt' dictionary using the 'test' function.
print("\nCount the frequency of the said dictionary:")
print(test(dictt))
Sample Output:
Original Dictionary: {'V': 10, 'VI': 10, 'VII': 40, 'VIII': 20, 'IX': 70, 'X': 80, 'XI': 40, 'XII': 20} Count the frequency of the said dictionary: Counter({10: 2, 40: 2, 20: 2, 70: 1, 80: 1})
Flowchart:
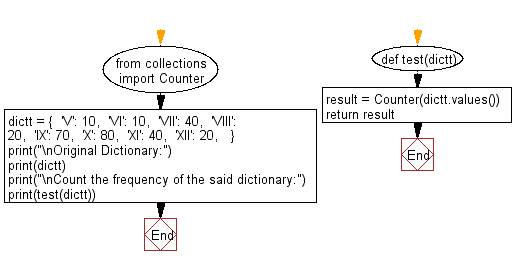
For more Practice: Solve these Related Problems:
- Write a Python program to count the frequency of each value in a dictionary using collections.Counter.
- Write a Python program to iterate over dictionary values and build a new dictionary mapping each value to its occurrence count.
- Write a Python program to use dictionary comprehension to generate a frequency dictionary from the values of another dictionary.
- Write a Python program to implement a function that returns the frequency distribution of dictionary values.
Go to:
Previous: Write a Python program to find shortest list of values with the keys in a given dictionary
Next: Write a Python program to extract values from a given dictionaries and create a list of lists from those values.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.