Python: Invert a given dictionary with non-unique hashable values
Python dictionary: Exercise-67 with Solution
Write a Python program to invert a given dictionary with non-unique hashable values.
- Create a collections.defaultdict with list as the default value for each key.
- Use dictionary.items() in combination with a loop to map the values of the dictionary to keys using dict.append().
- Use dict() to convert the collections.defaultdict to a regular dictionary.
Sample Solution:
Python Code:
# Import the 'defaultdict' class from the 'collections' module.
from collections import defaultdict
# Define a function 'test' that takes a dictionary 'students' as an argument.
def test(students):
# Create a 'defaultdict' object 'obj' with a default value of an empty list.
obj = defaultdict(list)
# Iterate through the items (key-value pairs) in the 'students' dictionary.
for key, value in students.items():
# Append the 'key' to the list associated with the 'value' in the 'obj' dictionary.
obj[value].append(key)
# Convert the 'defaultdict' 'obj' back to a regular dictionary and return it.
return dict(obj)
# Define a dictionary 'students' with student names as keys and grades as values.
students = {
'Ora Mckinney': 8,
'Theodore Hollandl': 7,
'Mae Fleming': 7,
'Mathew Gilbert': 8,
'Ivan Little': 7,
}
# Call the 'test' function with the 'students' dictionary and print the result.
print(test(students))
Sample Output:
{8: ['Ora Mckinney', 'Mathew Gilbert'], 7: ['Theodore Hollandl', 'Mae Fleming', 'Ivan Little']}
Flowchart:
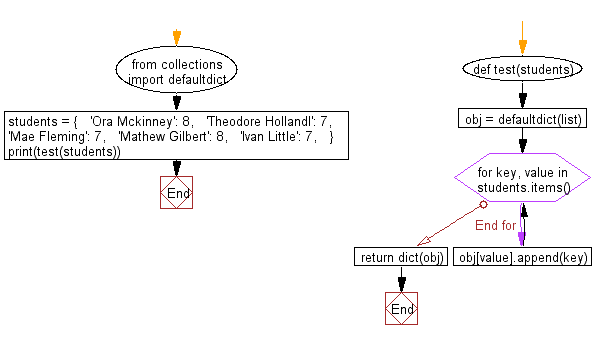
Python Code Editor:
Previous: Write a Python program to check if a specific Key and a value exist in a dictionary.
Next: Write a Python program to combines two or more dictionaries, creating a list of values for each key.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/dictionary/python-data-type-dictionary-exercise-67.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics