Python: Invert a dictionary with unique hashable values
Write a Python program to invert a dictionary with unique hashable values.
- Use dictionary.items() in combination with a list comprehension to create a new dictionary with the values and keys inverted.
Sample Solution:
Python Code:
# Define a function 'test' that takes a dictionary 'students'.
def test(students):
# Create a new dictionary where the keys and values from 'students' are swapped.
# The values become keys, and the keys become values in the new dictionary.
return {value: key for key, value in students.items()}
# Create a dictionary 'students' with names as keys and corresponding grades as values.
students = {
'Theodore': 10,
'Mathew': 11,
'Roxanne': 9,
}
# Call the 'test' function with the 'students' dictionary to swap keys and values.
print(test(students))
Sample Output:
{10: 'Theodore', 11: 'Mathew', 9: 'Roxanne'}
Flowchart:
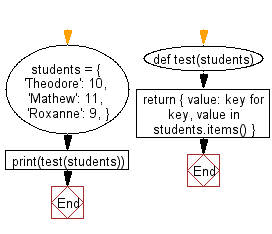
Python Code Editor:
Previous: Write a Python program to retrieve the value of the nested key indicated by the given selector list from a dictionary or list.
Next: Write a Python program to convert a list of dictionaries into a list of values corresponding to the specified key.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics