Python: Create a flat list of all the keys in a flat dictionary
78. Create Flat List of All Keys in a Dictionary
Write a Python program to create a flat list of all the keys in a flat dictionary.
- Use dict.keys() to return the keys in the given dictionary.
- Return a list() of the previous result.
Sample Solution:
Python Code:
# Define a function 'test' that takes a dictionary 'flat_dict'.
# The function returns a list of keys from the given dictionary.
def test(flat_dict):
# Use the 'keys' method to extract all the keys from the dictionary and convert them to a list.
return list(flat_dict.keys())
# Create a dictionary 'students' with key-value pairs.
students = {
'Theodore': 19,
'Roxanne': 20,
'Mathew': 21,
'Betty': 20
}
# Print the original dictionary.
print("\nOriginal dictionary elements:")
print(students)
# Call the 'test' function to create a flat list of all the keys in the 'students' dictionary.
print("\nCreate a flat list of all the keys of the said flat dictionary:")
print(test(students))
Sample Output:
Original dictionary elements: {'Theodore': 19, 'Roxanne': 20, 'Mathew': 21, 'Betty': 20} Create a flat list of all the keys of the said flat dictionary: ['Theodore', 'Roxanne', 'Mathew', 'Betty']
Flowchart:
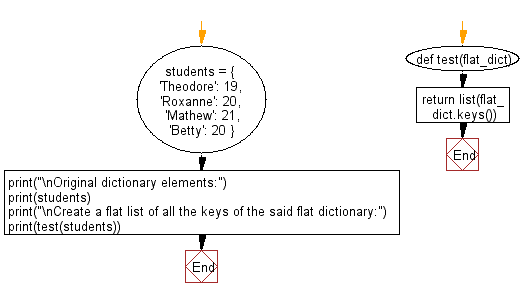
For more Practice: Solve these Related Problems:
- Write a Python program to extract all keys from a flat dictionary and store them in a list using the keys() method.
- Write a Python program to iterate over a dictionary and build a list of keys manually.
- Write a Python program to use list comprehension to create a list of all dictionary keys.
- Write a Python program to implement a function that returns a sorted list of keys from a dictionary.
Go to:
Previous: Write a Python program to convert given a dictionary to a list of tuples.
Next: Write a Python program to create a flat list of all the values in a flat dictionary.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.