Python program to convert string to bytes using different Encodings
1. String to Bytes Conversion
Write a Python program to convert a given string to bytes using different encodings like UTF-8, UTF-16, ASCII etc.
Sample Solution:
Code:
def convert_to_bytes(input_string, encoding):
try:
encoded_bytes = input_string.encode(encoding)
return encoded_bytes
except Exception as e:
print("Error:", e)
return None
def main():
try:
input_string = "Python Exercises!"
encodings = ["utf-8", "utf-16", "ascii"]
for encoding in encodings:
encoded_bytes = convert_to_bytes(input_string, encoding)
if encoded_bytes is not None:
print(f"Encoding: {encoding.upper()}")
print("Original String:", input_string)
print("Encoded Bytes:", encoded_bytes)
print("Decoded String:", encoded_bytes.decode(encoding))
print("=" * 40)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Encoding: UTF-8 Original String: Python Exercises! Encoded Bytes: b'Python Exercises!' Decoded String: Python Exercises!
Encoding: UTF-16 Original String: Python Exercises! Encoded Bytes: b'\xff\xfeP\x00y\x00t\x00h\x00o\x00n\x00 \x00E\x00x\x00e\x00r\x00c\x00i\x00s\x00e\x00s\x00!\x00' Decoded String: Python Exercises!
Encoding: ASCII Original String: Python Exercises! Encoded Bytes: b'Python Exercises!' Decoded String: Python Exercises!
In the exercise above, the "convert_to_bytes()" function takes an input string and an encoding as arguments and encodes the string using the provided encoding. In the "main()" function, the input string is converted to different encodings (UTF-8, UTF-16, ASCII). It then decodes the encoded bytes back to strings and prints the results.
Flowchart:
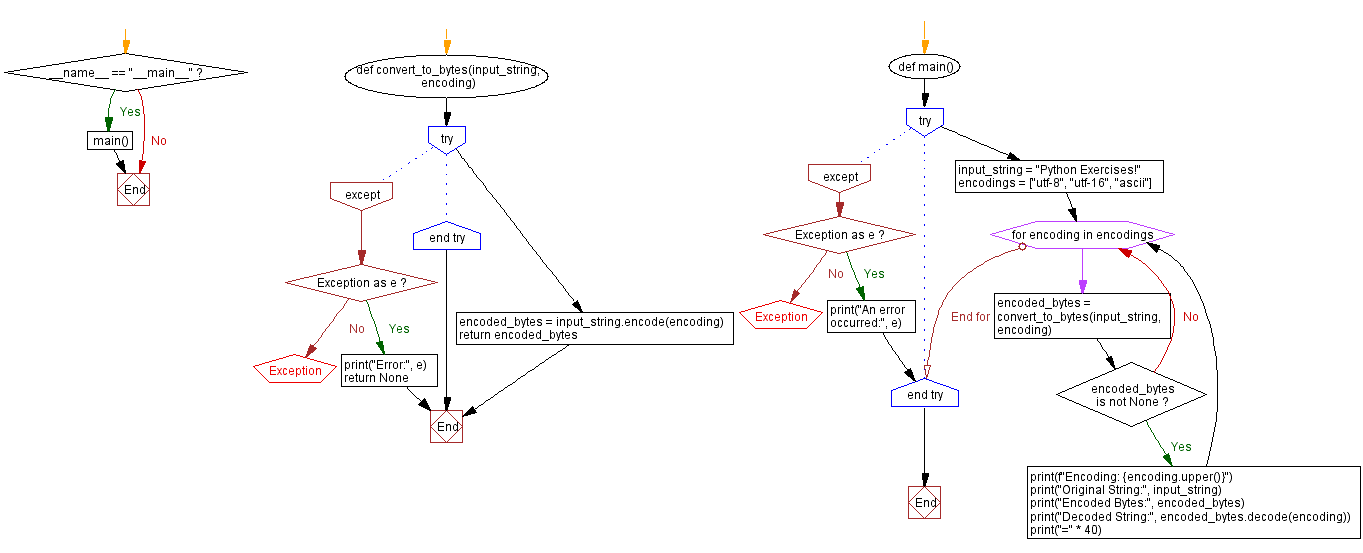
For more Practice: Solve these Related Problems:
- Write a Python program to convert a given string to bytes using UTF-8 encoding and then decode it back to a string.
- Write a Python script to encode a string to bytes using UTF-16 and display the byte values in hexadecimal format.
- Write a Python function that converts a string to bytes using ASCII encoding while handling encoding errors gracefully.
- Write a Python program to compare the lengths of the byte representations of a string in UTF-8, UTF-32, and Latin-1 encodings.
Go to:
Python Code Editor :
Previous: Python Extended Data Type Bytes and Byte Arrays Exercises Home.
Next: Python program for concatenating bytes objects
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.