Python Program: Check equality of bytes objects
Python Bytes and Byte Arrays Data Type: Exercise-7 with Solution
Write a Python program that checks if two bytes objects are equal.
Sample Solution:
Code:
def are_bytes_equal(bytes_obj1, bytes_obj2):
return bytes_obj1 == bytes_obj2
def main():
try:
bytes_obj1 = b"Python"
bytes_obj2 = b"Java"
bytes_obj3 = b"Exercises"
bytes_obj4 = b"Python"
are_equal_obj1_obj2 = are_bytes_equal(bytes_obj1, bytes_obj2)
are_equal_obj1_obj3 = are_bytes_equal(bytes_obj1, bytes_obj3)
are_equal_obj1_obj4 = are_bytes_equal(bytes_obj1, bytes_obj4)
print("Bytes Object-1:", bytes_obj1)
print("Bytes Object-2:", bytes_obj2)
print("Bytes Object-3:", bytes_obj3)
print("\nAre Bytes Object-1 and Object-2 equal?", are_equal_obj1_obj2)
print("Are Bytes Object-1 and Object-3 equal?", are_equal_obj1_obj3)
print("Are Bytes Object-1 and Object-4 equal?", are_equal_obj1_obj4)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Bytes Object-1: b'Python' Bytes Object-2: b'Java' Bytes Object-3: b'Exercises' Are Bytes Object-1 and Object-2 equal? False Are Bytes Object-1 and Object-3 equal? False Are Bytes Object-1 and Object-4 equal? True
In the exercise above, the "are_bytes_equal()" function takes two bytes objects as input and compares them using the equality operator '=='. In the "main()" function, sample bytes objects are provided and their equality is checked.
Flowchart:
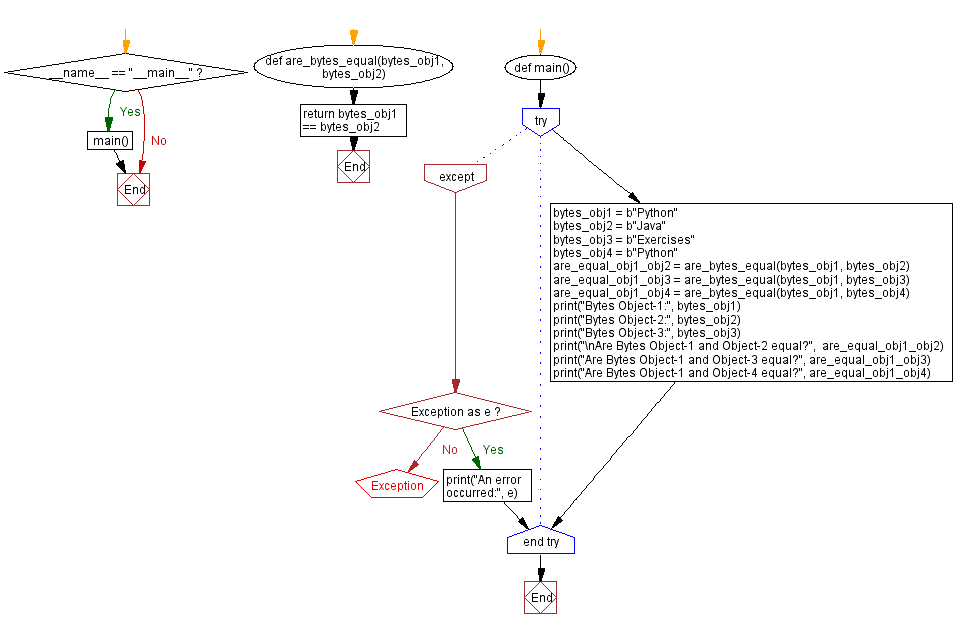
Previous: Reverse a given bytes object.
Next: Compress and decompress bytes using zlib
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/extended-data-types/python-extended-data-types-bytes-bytearrays-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics