Python Program: Compress and decompress bytes using zlib
8. Zlib Compression and Decompression
Write a Python program to compress and decompress a bytes sequence using zlib.
Sample Solution:
Code:
import zlib
def compress_bytes(byte_obj):
compressed_bytes = zlib.compress(byte_obj)
return compressed_bytes
def decompress_bytes(compressed_byte_obj):
decompressed_bytes = zlib.decompress(compressed_byte_obj)
return decompressed_bytes
def main():
try:
original_string = b"Python Exercises."
compressed_string = compress_bytes(original_string)
decompressed_string = decompress_bytes(compressed_string)
print("Original Bytes:", original_string)
print("\nCompressed Bytes:", compressed_string)
print("\nDecompressed Bytes:", decompressed_string)
print("\nDecompressed String:", decompressed_string.decode("utf-8"))
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Original Bytes: b'Python Exercises.' Compressed Bytes: b'x\x9c\x0b\xa8,\xc9\xc8\xcfSp\xadH-J\xce,N-\xd6\x03\x00;6\x06|' Decompressed Bytes: b'Python Exercises.' Decompressed String: Python Exercises.
In the above exercise the "compress_bytes()" function compresses a bytes sequence using the "zlib.compress()" function. The "decompress_bytes()" function decompresses the compressed bytes using the "zlib.decompress()" function. In the "main()" function, a sample sequence of bytes is compressed, decompressed, and the original, compressed, and decompressed results are printed.
Flowchart:
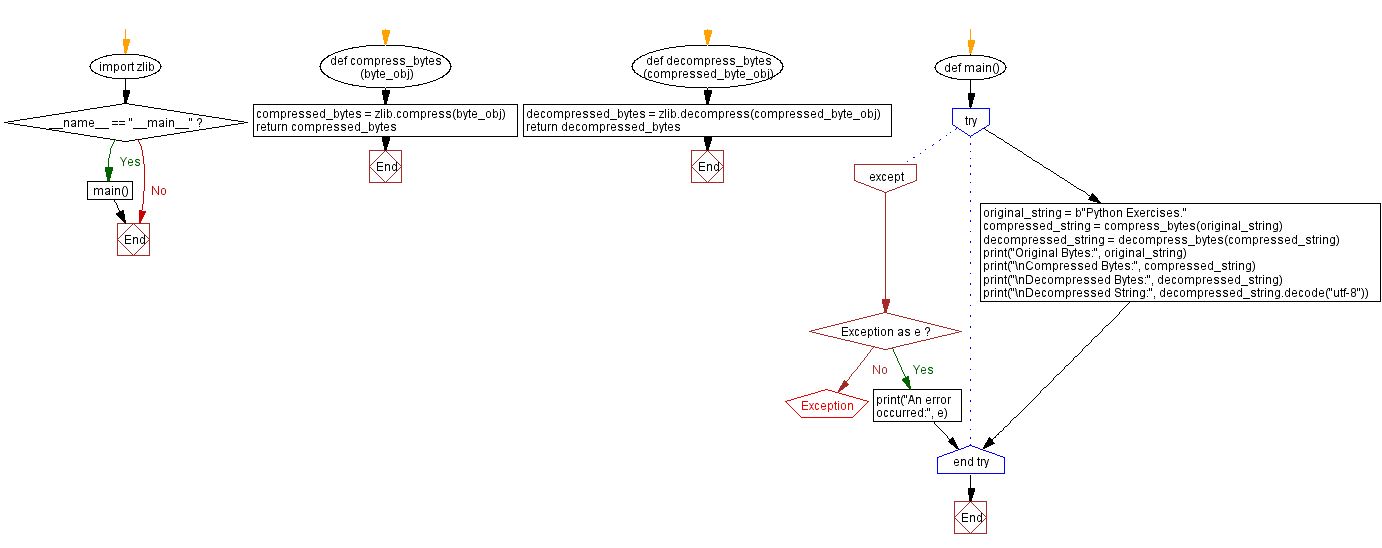
For more Practice: Solve these Related Problems:
- Write a Python program to compress a given bytes sequence using zlib and then decompress it back to the original bytes.
- Write a Python script to compare the size of a bytes sequence before and after zlib compression.
- Write a Python function to compress text converted to bytes using zlib, then decompress and decode it to verify the content.
- Write a Python program to compress a large bytes object with zlib and then measure the time taken for compression and decompression.
Go to:
Previous: Check equality of bytes objects.
Next: Search bytes sequence in file
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.