Python Function: Search bytes sequence in file
9. Search Bytes in File
Write a Python function that searches for a bytes sequence within a file opened in byte mode.
Sample Solution:
Code:
import io
def search_bytes_in_file(file_path, match_bytes):
with io.open(file_path, mode='rb') as file:
for line in file:
if match_bytes in line:
print(f"Match found in file {file_path}")
return True
else:
return False
# Example usage
match = b'foobar'
result = search_bytes_in_file('fontawesome-webfont.bin', match)
if result:
print("Match found")
else:
print("No match")
Output:
No match
Explanation:
In the exercise above -
- Open file in byte mode 'rb'
- Iterate over the file line-by-line as bytes
- Use the 'in' operator to check if match_bytes is present
- Return True if match found, False otherwise
- Can search any bytes sequence like encoded data
Flowchart:
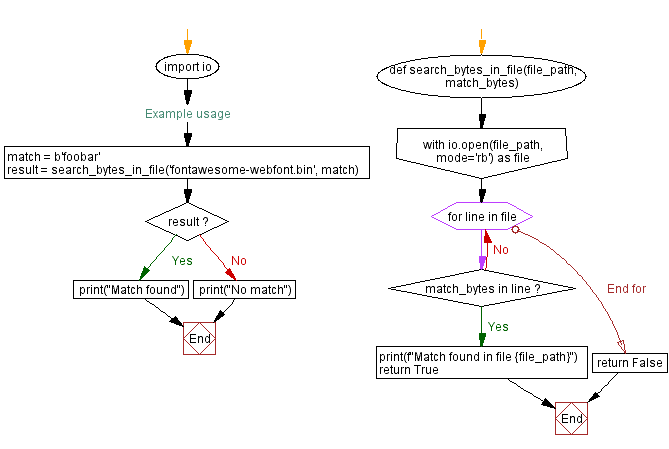
For more Practice: Solve these Related Problems:
- Write a Python function to open a file in binary mode and search for a specific bytes sequence, returning its starting index if found.
- Write a Python program to read a binary file and use a bytes search method to count the occurrences of a particular byte pattern.
- Write a Python script to scan a file opened in byte mode for a given bytes substring and then print all positions where it occurs.
- Write a Python program to search for a bytes sequence in a binary file and then output the surrounding context of the found sequence.
Go to:
Previous: Compress and decompress bytes using zlib.
Next: Convert bytearray to bytes
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.