Check even or odd number using Boolean variables in Python
Write a Python program to check if a given number is even or odd using boolean variables.
Sample Solution:
Code:
def is_even(number):
# Return True or False.
return number % 2 == 0
def main():
try:
number = int(input("Input a number: "))
if is_even(number):
print(f"{number} is an even number.")
else:
print(f"{number} is an odd number.")
except ValueError:
print("Invalid input. Please enter a valid number.")
if __name__ == "__main__":
main()
Output:
Input a number: 10 10 is an even number.
Input a number: 7 7 is an odd number.
When we run the program, it prompts the user to input a number. It then uses the “is_even()” function to determine if the number is even or odd and prints the appropriate message.
Flowchart:
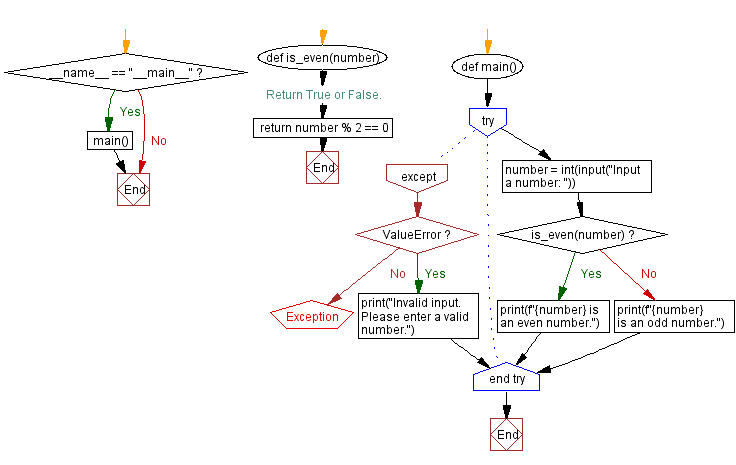
Previous: Python Extended Data Type Exercises Home.
Next: Python logical AND and OR operations with Boolean values
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics