Python list empty check using Boolean logic
Write a Python function that checks if a given list is empty or not using boolean logic.
Sample Solution:
Code:
def is_empty_list(lst):
return not bool(lst)
def main():
try:
input_list = eval(input("Input a list (e.g., [1, 2, 3, 4]): "))
if isinstance(input_list, list):
if is_empty_list(input_list):
print("The list is empty.")
else:
print("The list is not empty.")
else:
print("Invalid input. Please enter a valid list.")
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Input a list (e.g., [1, 2, 3, 4]): [1,2,3] The list is not empty.
Input a list (e.g., [1, 2, 3, 4]): [] The list is empty.
In the above exercise the program prompts the user to input a list (using eval to convert the input string to a list), and then uses the "is_empty_list()" function to determine if the list is empty or not. Based on the results, it prints the appropriate message.
Flowchart:
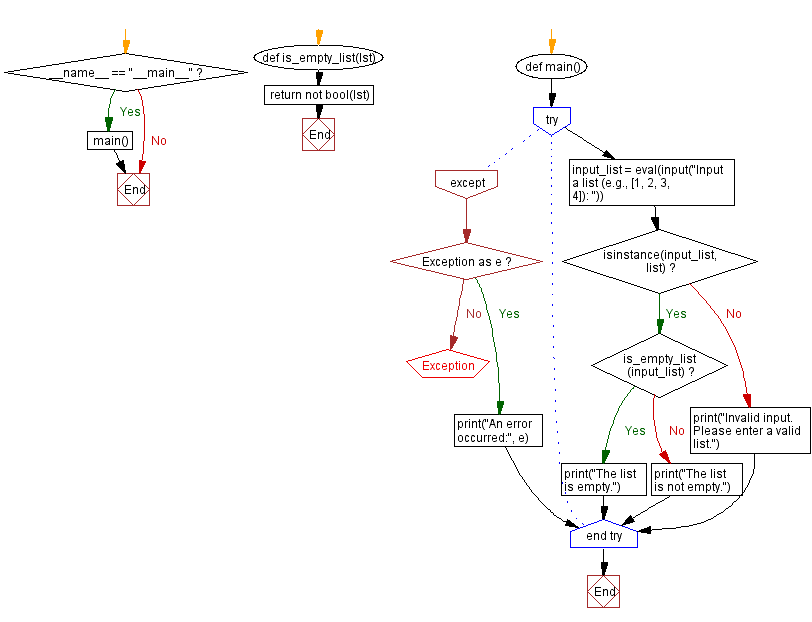
Previous: Voting eligibility checker.
Next: Python leap year checker using Boolean logic.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics