Python Program: Counting common elements in a list
2. Counter from List Elements
Write a Python program that creates a 'Counter' from a list of elements and print the most common elements along with their counts.
Sample Solution:
Code:
from collections import Counter
elements = [1, 2, 3, 4, 5, 11, 3, 3, 6, 7, 8, 9, 3, 10, 1]
element_counter = Counter(elements)
print("Most Common Elements:")
for element, count in element_counter.most_common():
print(f"{element}: {count}")
Output:
Most Common Elements: 3: 4 1: 2 2: 1 4: 1 5: 1 11: 1 6: 1 7: 1 8: 1 9: 1 10: 1
In the exercise above, the "Counter" class is used to count the occurrences of each element in the given list. The "most_common()" method retrieves the most common elements along with their counts in descending order. After iterating through the most common elements, the program prints their counts.
Flowchart:
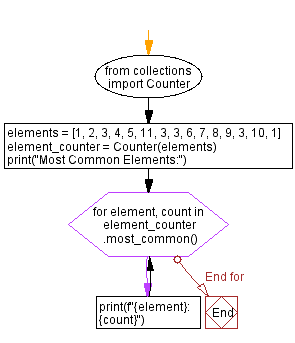
For more Practice: Solve these Related Problems:
- Write a Python program to create a Counter from a list of integers and then print the two most frequent numbers along with their counts.
- Write a Python script to build a Counter from a list of strings and then output all elements with a frequency less than 3.
- Write a Python function that takes a list of mixed elements, generates a Counter, and prints the frequency distribution in descending order.
- Write a Python program to convert a list of words into a Counter and then display the total number of unique words.
Go to:
Previous: Python Program: Counting letters in a string.
Next: Python Program: Counting vowels in a word.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.