Python Counter sum function: Add two counter objects
Write a function that takes two 'Counter' objects and returns their sum.
Sample Solution:
Code:
from collections import Counter
def add_counters(ctr1, ctr2):
return ctr1 + ctr2
def main():
counter1 = Counter({'item1': 10, 'item2': 34, 'item3': 22})
counter2 = Counter({'item1': 12, 'item2': 20, 'item3': 10})
result_counter = add_counters(counter1, counter2)
print("Counter 1:", counter1)
print("Counter 2:", counter2)
print("Sum Counter:", result_counter)
if __name__ == "__main__":
main()
Output:
Counter 1: Counter({'item2': 34, 'item3': 22, 'item1': 10}) Counter 2: Counter({'item2': 20, 'item1': 12, 'item3': 10}) Sum Counter: Counter({'item2': 54, 'item3': 32, 'item1': 22})
In the exercise above, the "add_counters()" function takes two "Counter" objects as input and returns their sum. By using the '+' operator, both counters are added element-by-element, which combines the common and unique counts of both counters.
Flowchart:
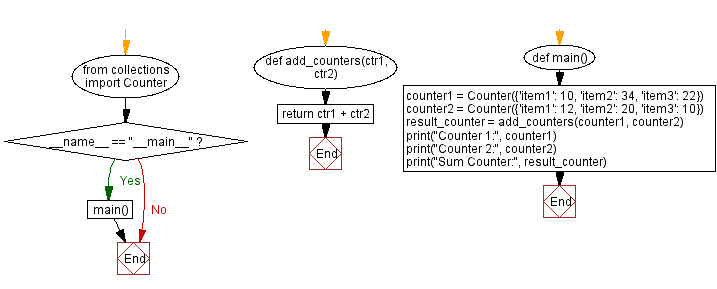
Previous: Python Program: Counting and sorting word frequencies.
Next: Python Program: Convert counter to list of unique Items.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics