Python Program: Convert counter to list of unique Items
Write a Python program that creates a 'Counter' for a list of items and converts it to a list of unique items with their counts.
Sample Solution:
Code:
from collections import Counter
def main():
items = ["Red", "Green", "Black", "Black", "Red", "red", "Orange", "Pink", "Pink", "Red", "White"]
item_counter = Counter(items)
unique_items_with_counts = list(item_counter.items())
print("Original List:", items)
print("Counter:", item_counter)
print("Unique Items with Counts:", unique_items_with_counts)
if __name__ == "__main__":
main()
Output:
Counter 1: Counter({'item2': 34, 'item3': 22, 'item1': 10}) Counter 2: Counter({'item2': 20, 'item1': 12, 'item3': 10}) Sum Counter: Counter({'item2': 54, 'item3': 32, 'item1': 22})
In the exercise above, the "Counter" class is used to create a counter for the list of items. The "items()" method of the 'Counter' object retrieves a list of distinct items along with their counts, which is then printed.
Flowchart:
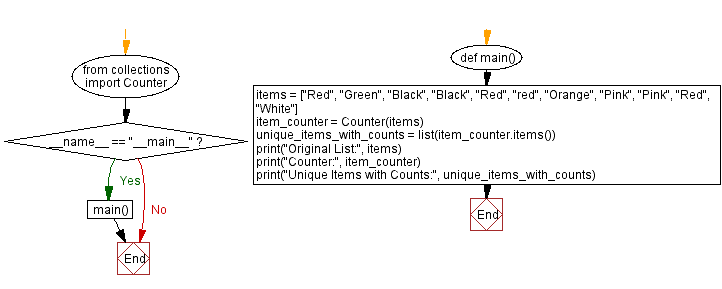
Previous: Python Counter sum function: Add two counter objects.
Next: Python counter filter program: Counting and filtering words.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics