Python Program: Checking and handling ellipsis in variables
5. Ellipsis Equality Checker
Write a Python program that checks if a variable is equal to ... and prints a message if it matches.
Sample Solution:
Code:
# Define a list with ellipsis as a placeholder
nums = [1, 2, 3, 4, 5, 6, ...]
for item in nums:
if item is ...:
print("Skipping...")
continue
print(f"Reading item: {item}")
Output:
Reading item: 1 Reading item: 2 Reading item: 3 Reading item: 4 Reading item: 5 Reading item: 6 Skipping...
The exercise above defines a list 'nums' with 'ellipsis' as a placeholder for unspecified items. In the loop, we check if the current item is 'ellipsis (...)', and if it is, we print "Skipping...". Otherwise, we process the item and print a message indicating that we are processing it. By using 'ellipsis' as a marker for skipped or unspecified items, we can iterate over an unknown number of items.
Flowchart:
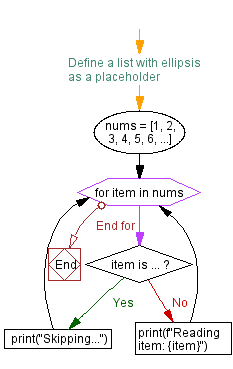
For more Practice: Solve these Related Problems:
- Write a Python program to define a variable as Ellipsis and check if another variable is exactly Ellipsis using the 'is' operator, then print the result.
- Write a Python function that takes a variable and returns True if it is Ellipsis, otherwise returns False, and test it with various inputs.
- Write a Python script to iterate through a list of mixed elements and print a message for each element that is exactly Ellipsis.
- Write a Python program to create a list containing several values including Ellipsis and then filter out all elements that are equal to Ellipsis.
Go to:
Previous: Python Program: Creating lists with gaps using ellipsis.
Next: Python: Creating multidimensional arrays with unspecified dimensions.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.