Generating sequences with ellipsis in Python
7. Generator with Ellipsis Gap
Write a Python program to create a generator expression that generates a sequence of numbers with ellipsis representing skipped values.
Sample Solution:
Code:
def generate_sequence(start, end, step, skip_count):
"""
Args:
start (int): The starting value of the sequence.
end (int): The ending value of the sequence.
step (int): The step size between values.
skip_count (int): The number of values to skip with ellipsis.
Returns:
A generator expression for the sequence.
"""
for i in range(start, end, step):
if skip_count > 0:
yield i
skip_count -= 1
else:
yield "..."
skip_count = skip_count + step - 1
sequence_generator = generate_sequence(1, 30, 2, 4)
for item in sequence_generator:
print(item, end=", ")
Output:
1, 3, 5, 7, ..., 11, ..., 15, ..., 19, ..., 23, ..., 27, ...,
In the exercise above, the "generate_sequence()" function takes parameters for the starting value ('start'), ending value ('end'), step size ('step'), and the number of values to skip with 'ellipsis' (skip_count). It uses a generator expression to yield values from 'start' to 'end' with the specified step size. When it reaches 'skip_count', it yields 'ellipsis (...)' to represent skipped values.
Flowchart:
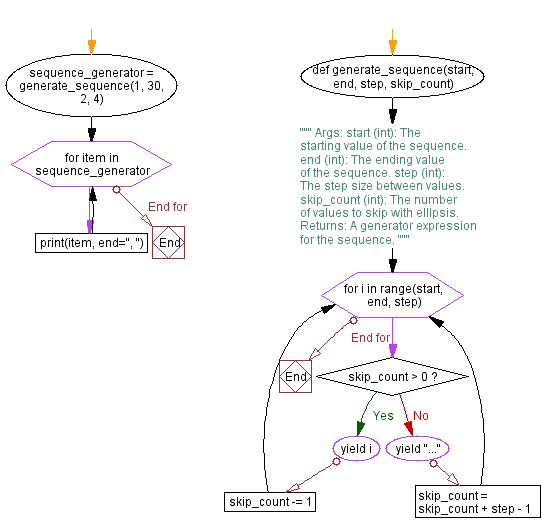
For more Practice: Solve these Related Problems:
- Write a Python program to create a generator expression that yields numbers from 1 to 20, but yields Ellipsis between 10 and 15 to indicate a gap.
- Write a Python function that returns a generator yielding the first 5 numbers, then an Ellipsis, followed by the last 5 numbers from a specified range.
- Write a Python script to build a generator expression that outputs a sequence with Ellipsis inserted if the gap between successive numbers exceeds 1.
- Write a Python program to implement a generator that yields numbers from a range and yields Ellipsis when the current number is divisible by a specific value.
Go to:
Previous: Python: Creating multidimensional arrays with unspecified dimensions.
Next: Extending sequences with ellipsis in Python.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.