Extending sequences with ellipsis in Python
8. Extend Sequence with Ellipsis
Write a Python program that uses Ellipsis to extend a sequence with another sequence.
Sample Solution:
Code:
# Define three sequences
sequence1 = [1, 2, 3]
sequence2 = [4, 5, 6]
sequence3 = [7, 8, 9]
# Extend sequence1, sequence2 and sequence3 using ellipsis
extended_sequence = sequence1 + [...] + sequence2 + [...] + sequence3
# Print the extended sequence
print(extended_sequence)
Output:
[1, 2, 3, Ellipsis, 4, 5, 6, Ellipsis, 7, 8, 9]
In the exercise above, we have three sequences, sequence1, sequence2 and sequence3. We use 'ellipsis (...)' between them to represent an extension operation. The result, extended_sequence, will contain the elements from sequence1, sequence2 and sequence3 concatenated together.
Flowchart:
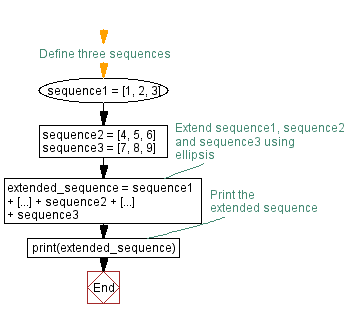
For more Practice: Solve these Related Problems:
- Write a Python program to extend a list by using Ellipsis as a marker to indicate where the new sequence should be inserted, then merge the two sequences.
- Write a Python script to combine two sequences where Ellipsis in the first sequence indicates the insertion point for the second sequence.
- Write a Python function that takes two lists and uses Ellipsis to splice the second list into the first at a designated position.
- Write a Python program to simulate sequence extension by using Ellipsis to represent missing data, then replace Ellipsis with values from another list.
Go to:
Previous: Generating sequences with ellipsis in Python.
Next: Creating Python classes with dynamic attributes.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.