Python frozen set and set conversion: Differences and comparisons
2. Frozenset and Set Conversion
Write a Python program that converts a "frozen set" to a regular set and vice versa. Compare the differences between these two data types.
Sample Solution:
Code:
frozen_set = frozenset([1, 2, 3, 4, 5])
regular_set = set([3, 4, 5, 6, 7])
# Convert frozen set to regular set
converted_to_set = set(frozen_set)
# Convert regular set to frozen set
converted_to_frozenset = frozenset(regular_set)
print("Frozen Set:", frozen_set)
print("Type of Frozen Set:", type(frozen_set))
print("\nConverted to Set:", converted_to_set)
print("Type of Converted Set:", type(converted_to_set))
print("\nRegular Set:", regular_set)
print("Type of Regular Set:", type(regular_set))
print("\nConverted to Frozen Set:", converted_to_frozenset)
print("Type of Converted Frozen Set:", type(converted_to_frozenset))
Output:
Frozen Set: frozenset({1, 2, 3, 4, 5}) Type of Frozen Set: <class 'frozenset'> Converted to Set: {1, 2, 3, 4, 5} Type of Converted Set: <class 'set'> Regular Set: {3, 4, 5, 6, 7} Type of Regular Set: <class 'set'> Converted to Frozen Set: frozenset({3, 4, 5, 6, 7}) Type of Converted Frozen Set: <class 'frozenset'>
Flowchart:
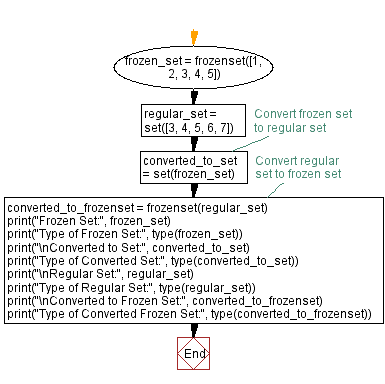
For more Practice: Solve these Related Problems:
- Write a Python program to convert a frozenset to a set, modify the set by adding a new element, then convert it back to a frozenset and print both.
- Write a Python function that takes a list, converts it to a set to remove duplicates, then to a frozenset, and finally back to a set, printing the type and content at each step.
- Write a Python script to compare the performance of membership tests in a set versus a frozenset by converting between the two and timing operations.
- Write a Python program to illustrate immutability by converting a mutable set to a frozenset, attempting to modify it, and then catching the expected exception.
Go to:
Previous: Python Frozenset set operations: Union, intersection, difference.
Next: Python frozenset symmetric difference: Function and example.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.