Frozenset immutability demonstration: Python code example
8. Frozenset Immutability Test
Write a Python program that demonstrates the immutability of a frozenset by attempting to add or remove elements from it.
Sample Solution:
Code:
def main():
original_frozenset = frozenset([1, 2, 3, 4, 5, 6])
# Attempt to add an element to the frozenset
try:
modified_frozenset = original_frozenset.add(7)
except AttributeError:
print("It is not possible to add an element to a frozenset!")
# Attempt to remove an element from the frozenset
try:
modified_frozenset = original_frozenset.remove(2)
except AttributeError:
print("It is not possible to remove an element from a frozenset!")
if __name__ == "__main__":
main()
Output:
It is not possible to add an element to a frozenset! It is not possible to remove an element from a frozenset!
In the exercise above, we are trying to use the "add()" and "remove()" methods to modify the original_frozenset. However, both of these operations will raise an "AttributeError" because a 'frozenset' does not have these methods due to its immutability.
Flowchart:
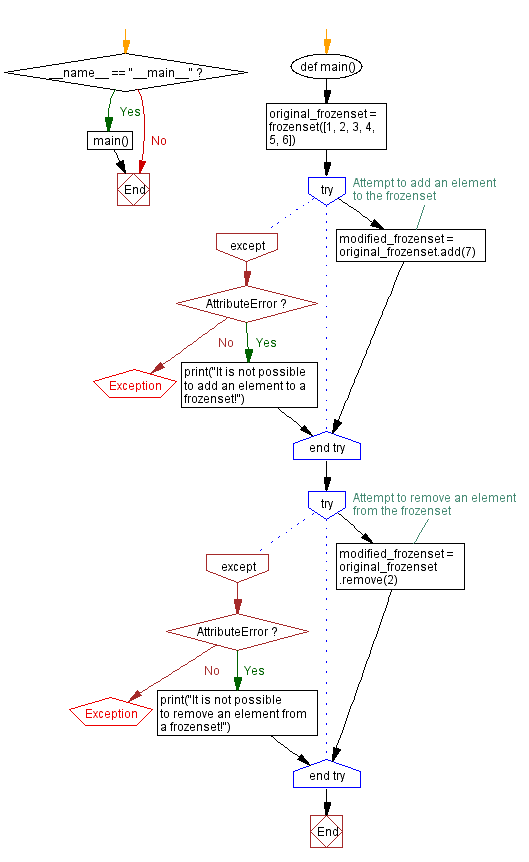
For more Practice: Solve these Related Problems:
- Write a Python program to attempt adding an element to a frozenset, then catch and display the resulting exception message.
- Write a Python script to demonstrate that removing an element from a frozenset is not allowed by triggering and handling a TypeError.
- Write a Python program to show the immutability of a frozenset by using it as a key in a dictionary and then attempting to modify it.
- Write a Python function that tries to modify a frozenset and returns a custom error string indicating that the operation is not permitted.
Go to:
Previous: Check disjoint frozensets: Python code and example.
Next: Hashable composite keys using frozenset: Python code example.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.