Hashable composite keys using frozenset: Python code example
9. Frozenset as Dictionary Key
Write a Python program that uses a frozenset as a key in a dictionary to create a hashable composite key for more complex data structures.
Sample Solution:
Code:
def main():
emp1 = {"name": "Bernardetta Frigg", "age": 20, "country": "Spain"}
emp2 = {"name": "Fadhili Lilah", "age": 22, "country": "France"}
emp3 = {"name": "Abbe Ivan", "age": 21, "country": "USA"}
employees_data = {
frozenset(emp1.items()): emp1,
frozenset(emp2.items()): emp2,
frozenset(emp3.items()): emp3
}
for key, value in employees_data.items():
print("Key:", key)
print("Value:", value)
print()
if __name__ == "__main__":
main()
Output:
Key: frozenset({('name', 'Bernardetta Frigg'), ('country', 'Spain'), ('age', 20)}) Value: {'name': 'Bernardetta Frigg', 'age': 20, 'country': 'Spain'} Key: frozenset({('age', 22), ('country', 'France'), ('name', 'Fadhili Lilah')}) Value: {'name': 'Fadhili Lilah', 'age': 22, 'country': 'France'} Key: frozenset({('country', 'USA'), ('age', 21), ('name', 'Abbe Ivan')}) Value: {'name': 'Abbe Ivan', 'age': 21, 'country': 'USA'}
In the exercise above, we have a dictionary 'employees_data' that uses frozenset instances as keys. Each frozenset key is created from the "items()" function of a dictionary representing employee data, where each employee's name, age and country are stored.
The program prints each key-value pair from the 'employees_data' dictionary. As a result of using frozenset keys, we create hashable composite keys that can uniquely identify complex data structures, such as dictionaries.
Flowchart:
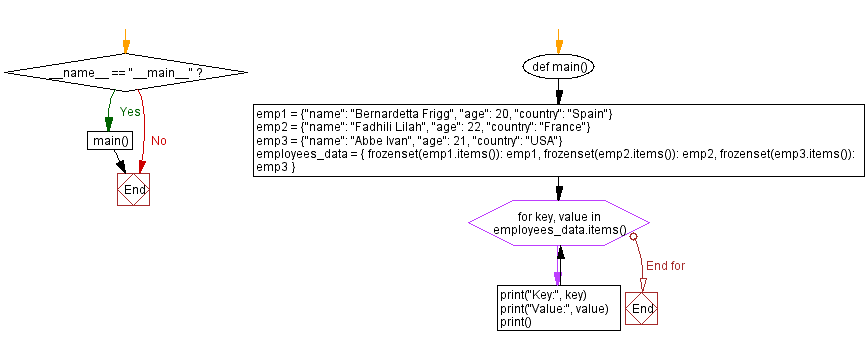
For more Practice: Solve these Related Problems:
- Write a Python program to use a frozenset as a key in a dictionary, mapping it to a complex data structure, and then retrieve the value.
- Write a Python script to create a dictionary with frozenset keys derived from lists and print the dictionary to verify hashability.
- Write a Python function that generates composite keys from multiple sets by converting them to frozensets and then uses them in a dictionary lookup.
- Write a Python program to demonstrate how frozensets can serve as dictionary keys by storing and retrieving information based on frozenset keys.
Python Code Editor :
Previous: Frozenset immutability demonstration: Python code example.
Next: Python program: Frozenset of squares of odd numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.