Creating a memory view on a Python bytes object: Length and first bytes
Write a Python program that creates a memory view on a bytes object and prints the length and first 8 bytes.
Sample Solution:
Code:
def main():
try:
data = b"Python Exercises!"
# Create memory view on data
mem_data = memoryview(data)
print("Memory View Length:", len(mem_data))
# Print first 8 bytes
print("First 8 Bytes:", mem_data[:8])
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Memory View Length: 17 First 8 Bytes: <memory at 0x00000266DBB4E1C8>
In the exercise above, we create a memory view named 'mem_data' on the 'data' bytes object using the "memoryview()" function. We then print the length of the memory view using the "len()" function and the first 8 bytes using slicing on the memory view.
Flowchart:
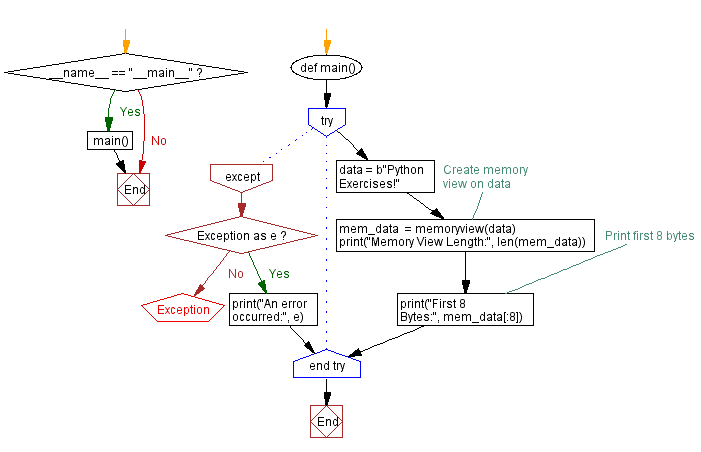
Previous: Python Extended Data Type Memory Views Exercises Home.
Next: Converting Python memory view to bytes: Function and example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics