Converting Python memory view to bytes: Function and example
2. Memory View to Bytes Conversion
Write a Python function that takes a memory view and converts it to a bytes object.
Sample Solution:
Code:
def memoryview_to_bytes(mem_view):
try:
# Convert memory view to bytes using bytes()
bytes_data = bytes(mem_view)
return bytes_data
except Exception as e:
print("An error occurred:", e)
return None
def main():
try:
data = b"Python Exercises!"
mem_view = memoryview(data)
print("Memory views: ",mem_view)
# Convert memory view to bytes
bytes_data = memoryview_to_bytes(mem_view)
if bytes_data is not None:
print("Bytes Object:", bytes_data)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Memory views: <memory at 0x00000266DBB4E108> Bytes Object: b'Python Exercises!'
The above exercise demonstrates how to convert a memory view to a bytes object using the bytes() constructor.
Flowchart:
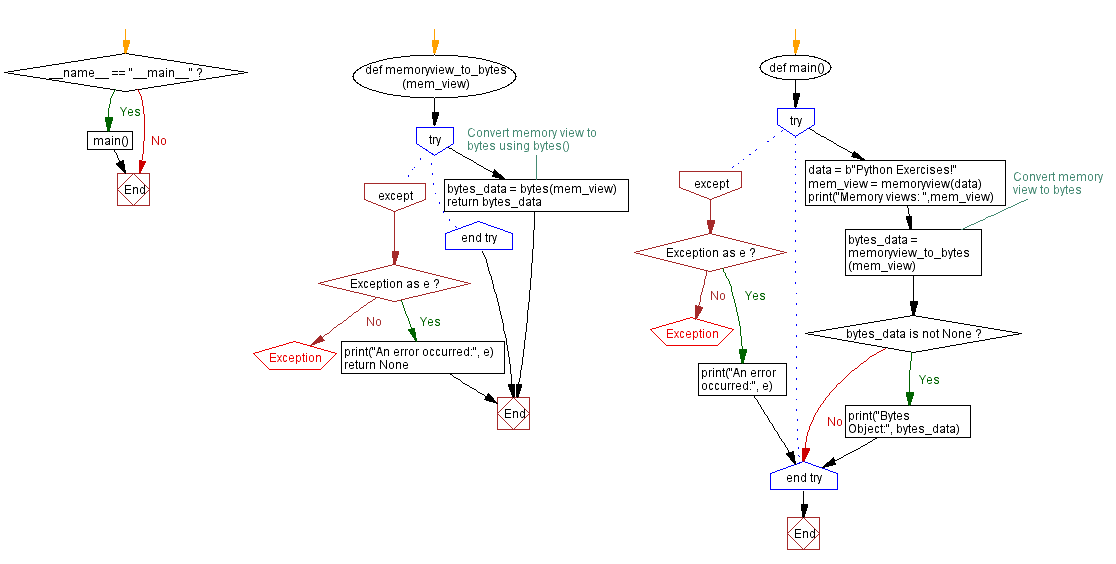
For more Practice: Solve these Related Problems:
- Write a Python function that accepts a memory view and converts it back to a bytes object, then prints the resulting bytes in both string and hexadecimal forms.
- Write a Python program to create a memory view on a bytes object, convert it back to bytes, and verify that the original and converted objects are identical.
- Write a Python script that takes a memory view sliced from a larger bytes object, converts the slice back to bytes, and displays its length.
- Write a Python function to accept a memory view, modify a copy of it, convert the copy to bytes, and compare it with the original bytes object.
Go to:
Previous: Creating a memory view on a Python bytes object: Length and first bytes.
Next: Creating NumPy memory views: 1D and 3D array examples in Python.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.