Creating NumPy memory views: 1D and 3D array examples in Python
3. 1D and 3D Memory View from NumPy Array
Write a Python program that creates a 1-dimensional and 3-dimensional memory view from a NumPy array.
Sample Solution:
Code:
import numpy as np
def main():
try:
# Create a NumPy array
array_1_d = np.array([1, 2, 3, 4, 5, 6])
array_3_d = np.array([[[1, 2, 3], [4, 5, 6]], [[7, 8, 9], [10, 11, 12]]])
# Create memory views from the NumPy arrays
print("Memory views:")
mem_view_1_d = memoryview(array_1_d)
print(mem_view_1_d)
mem_view_3_d = memoryview(array_3_d)
print(mem_view_3_d)
# Print the memory views
print("\n1-D Memory View:")
print(mem_view_1_d.tolist())
print("\n3-D Memory View:")
print(mem_view_3_d.tolist())
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Memory views: <memory at 0x00000266DBB4E108> <memory at 0x00000266DBB085E8> 1-D Memory View: [1, 2, 3, 4, 5, 6] 3-D Memory View: [[[1, 2, 3], [4, 5, 6]], [[7, 8, 9], [10, 11, 12]]]
The above exercise demonstrates how to create memory views from NumPy arrays and print their contents.
Flowchart:
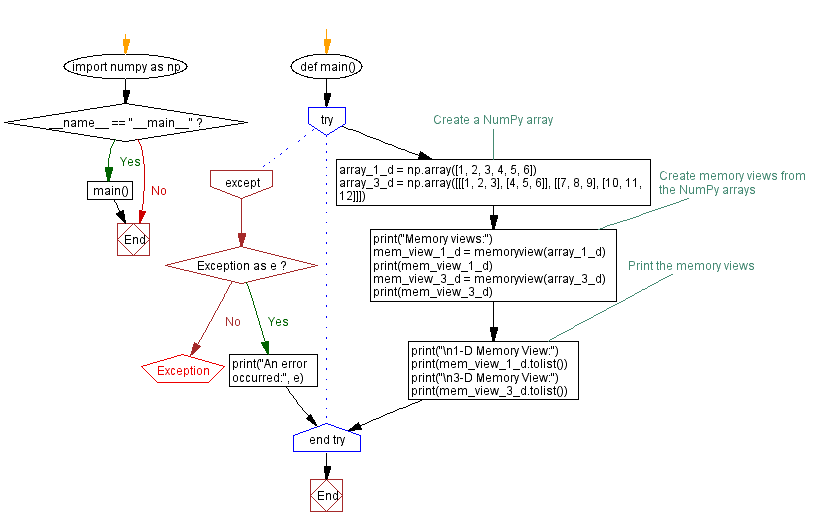
For more Practice: Solve these Related Problems:
- Write a Python program to create a 1-dimensional memory view from a NumPy array and print its shape and first few elements.
- Write a Python script to generate a 3-dimensional NumPy array, create a memory view, and then display the dimensions and a slice from the array.
- Write a Python function to convert a NumPy array into both a 1D and a 3D memory view, printing the type and shape of each view.
- Write a Python program to create a NumPy array, form a memory view of it in different dimensions, and verify that changes in the array are reflected in the memory view.
Go to:
Previous: Converting Python memory view to bytes: Function and example.
Next: Calculating average with NumPy memory view in Python.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.