Modifying binary files using Python memory views
5. Memory View on Binary File
Write a Python program that reads a binary file into a memory view and saves a modified copy.
Sample Solution:
Code:
def modify_file_data(data):
# Example modification: adding 10 to each byte
for i in range(len(data)):
data[i] = (data[i] + 10) % 256
def main():
input_file_path = "fontawesome_webfont.bin"
output_file_path = "output.bin"
# Read binary data from input file into a bytearray
with open(input_file_path, "rb") as input_file:
input_data = input_file.read()
input_bytearray = bytearray(input_data)
# Create a memory view from the bytearray
memory_view = memoryview(input_bytearray)
# Modify the data using the memory view
modify_file_data(memory_view)
# Save the modified data to an output file
with open(output_file_path, "wb") as output_file:
output_file.write(memory_view)
print("File modification complete.")
if __name__ == "__main__":
main()
Output:
File modification complete.
In the exercise above, the binary data is read from the input file into a 'bytearray', which is writable. Then, a memory view is created from this writable 'bytearray'. The "modify_file_data()" function modifies the bytearray data through the memory view. Finally, the modified data is written to an output file.
Flowchart:
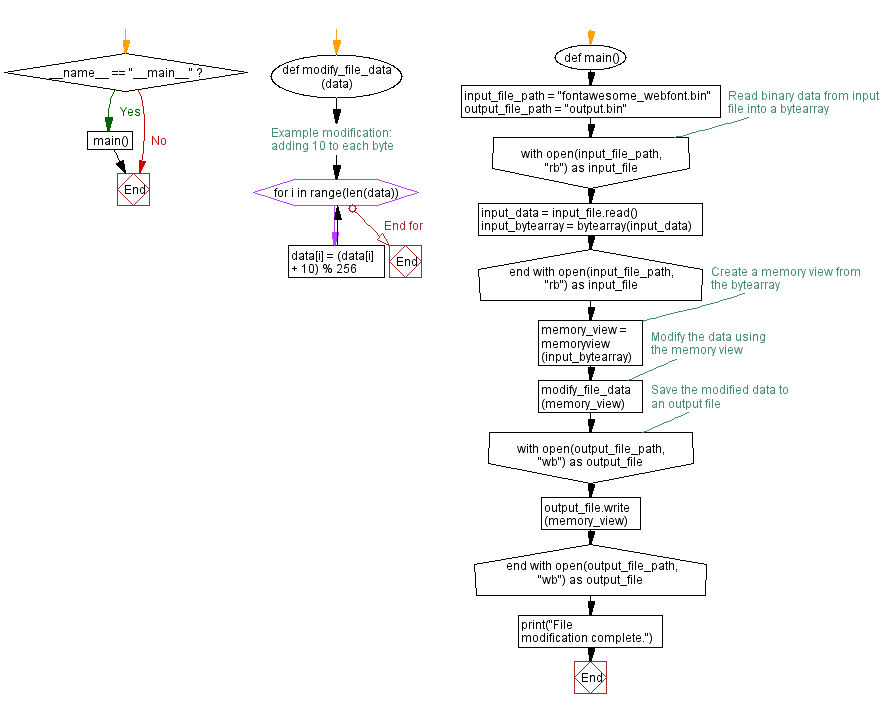
For more Practice: Solve these Related Problems:
- Write a Python program to open a binary file, create a memory view of its content, modify a specific byte, and then write the modified bytes back to a new file.
- Write a Python script to read a binary file into a memory view, change a range of bytes using slicing, and save the updated content to another file.
- Write a Python function that takes a binary file path, creates a memory view of its contents, and prints the first 16 bytes before and after modification.
- Write a Python program to load a binary file into a memory view, replace a specified byte pattern, and then save the altered bytes to a new file.
Go to:
Previous: Calculating average with NumPy memory view in Python.
Next: Concatenating memory views in Python: Function and example.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.