Concatenating memory views in Python: Function and example
Python Memory Views Data Type: Exercise-6 with Solution
Write a Python function that takes two memory views and concatenates them. Print the concatenated memory view.
Sample Solution:
Code:
def concatenate_memory_views(mem_view1, mem_view2):
result = bytearray(mem_view1) + bytearray(mem_view2)
return memoryview(result)
def main():
data1 = bytearray(b"Python ")
data2 = bytearray(b"Exercises!")
print("Memory views:")
memory_view1 = memoryview(data1)
print(memory_view1)
memory_view2 = memoryview(data2)
print(memory_view2)
concatenated_view = concatenate_memory_views(memory_view1, memory_view2)
print("Concatenated Memory View:", concatenated_view.tobytes().decode("utf-8"))
if __name__ == "__main__":
main()
Output:
Memory views: <memory at 0x000001A6784F7588> <memory at 0x000001A67988E108> Concatenated Memory View: Python Exercises!
In the above exercise, the "concatenate_memory_views()" function takes two memory views, converts them to bytearrays, concatenates them, and then returns a new memory view created from the concatenated bytearray.
The "main()" function creates two bytearrays, converts them to memory views, and then calls the "concatenate_memory_views()" function to concatenate them. In the end, it converts the concatenated memory view back to a string using the decode method with UTF-8 encoding.
Flowchart:
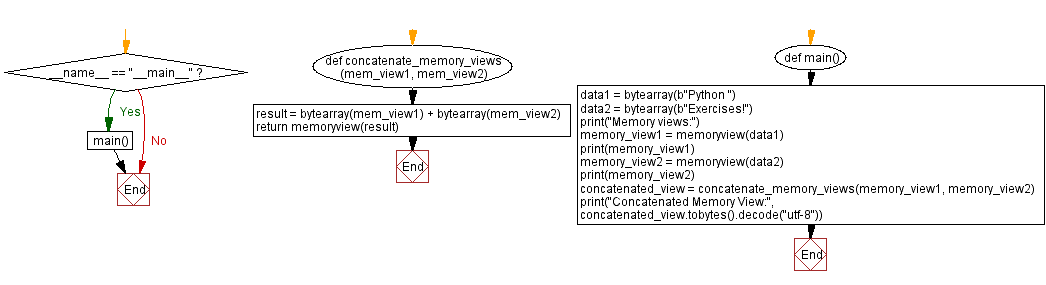
Previous: Modifying binary files using Python memory views.
Next: Iterating and modifying memory views in Python: Example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/extended-data-types/python-extended-data-types-index-memory-views-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics