Iterating and modifying memory views in Python: Example
7. Increment Memory View Elements
Write a Python program that iterates over a memory view and increment each element by 10 using a loop.
Sample Solution:
Code:
def test(memory_view):
for i in range(len(memory_view)):
memory_view[i] = (memory_view[i] + 10) % 256
def main():
data = bytearray([100, 200, 150, 200, 50])
memory_view = memoryview(data)
print("Original Memory View:", memory_view.tolist())
test(memory_view)
print("Modified Memory View:", memory_view.tolist())
if __name__ == "__main__":
main()
Output:
Original Memory View: [100, 200, 150, 200, 50] Modified Memory View: [110, 210, 160, 210, 60]
In the exercise above, the "test()" function takes a memory view as an argument and iterates over its elements using a loop. It increments each element by 10 and uses the modulo operation to ensure that the values stay within the valid range of 0 to 255. The "main()" function creates a bytearray, converts it to a memory view. Prints the original memory view, calls the "test()" function to modify the memory view, and then prints the modified memory view.
Flowchart:
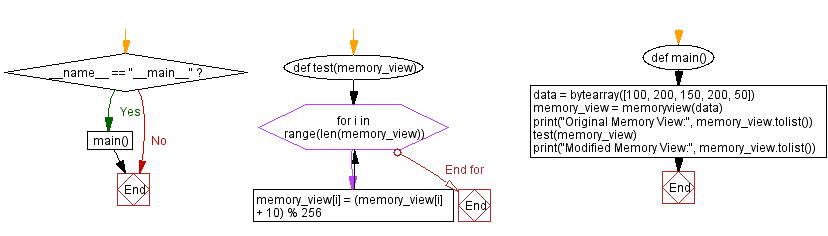
For more Practice: Solve these Related Problems:
- Write a Python program to iterate over a memory view of a bytearray and increment each element by 10, then print the modified bytearray.
- Write a Python function to loop through a memory view, add a constant value to each byte, and output the updated memory view as a list.
- Write a Python script to modify a memory view in place by increasing each element by 10 and then display the new values in both decimal and hexadecimal formats.
- Write a Python program to create a memory view from a mutable bytes object, increment each element by 10 using a for loop, and verify the changes reflect in the original object.
Go to:
Previous: Concatenating memory views in Python: Function and example.
Next: Reversing memory views in Python: Example and steps.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.