Reversing memory views in Python: Example and steps
8. Reverse Memory View from Bytearray
Write a Python program that creates a memory view from a bytearray, reverses the order using slicing, and converts back to a bytearray.
Sample Solution:
Code:
def test(memory_view):
reversed_memory_view = memory_view[::-1]
return bytearray(reversed_memory_view)
def main():
original_data = bytearray([10, 20, 30, 40, 50])
original_memory_view = memoryview(original_data)
print("Original Data:", original_data)
print("Original Memory View:", original_memory_view.tolist())
reversed_bytearray = test(original_memory_view)
print("Reversed Bytearray:", reversed_bytearray)
if __name__ == "__main__":
main()
Output:
Original Data: bytearray(b'\n\x14\x1e(2') Original Memory View: [10, 20, 30, 40, 50] Reversed Bytearray: bytearray(b'2(\x1e\x14\n')
In the exercise above, using slicing with the [::-1] notation, the "test()" function reverses the order of elements in a memory view, and then creates a new bytearray based on the reversed memory view.
The "main()" function creates an original bytearray, converts it to a memory view, and prints the original data and memory view. Then, it calls the "test()" function to reverse the memory view and convert it back to a bytearray . Finally, it prints the reversed bytearray.
Flowchart:
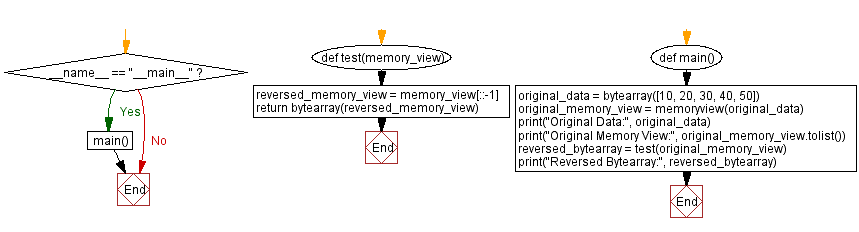
For more Practice: Solve these Related Problems:
- Write a Python program to create a memory view from a bytearray, reverse it using slicing, convert it back to a bytearray, and print the result.
- Write a Python function that takes a bytearray, creates a memory view, reverses the view, and returns the reversed bytearray.
- Write a Python script to demonstrate reversing a memory view created from a bytearray and then compare the reversed result with Python’s built-in reversed() function.
- Write a Python program to create a bytearray, form a memory view, reverse its order using slicing, and verify that the new bytearray contains the original elements in reverse order.
Python Code Editor :
Previous: Iterating and modifying memory views in Python: Example.
Next: Slicing memory views in Python: Indexing syntax and example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.