Python NamedTuple example: Employee information
1. Employee NamedTuple
Write a Python program that defines a NamedTuple called Employee with fields like name, age, and country. Print each employee's name and country.
Sample Solution:
Code:
from collections import namedtuple
# Define the NamedTuple Employee
Employee = namedtuple("Employee", ["name", "age", "country"])
def main():
try:
# Create a list of Employee instances
employees = [
Employee("Klaes Susana", 35, "USA"),
Employee("Auxentius Cloe", 44, "Canada"),
Employee("Golzar Merob", 28, "UK"),
Employee("Tatjana Adhelm", 30, "Australia"),
]
# Print each employee's name and country
for employee in employees:
print("Employee Name:", employee.name)
print("Employee Country:", employee.country)
print() # Print an empty line for separation
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Employee Name: Klaes Susana Employee Country: USA Employee Name: Auxentius Cloe Employee Country: Canada Employee Name: Golzar Merob Employee Country: UK Employee Name: Tatjana Adhelm Employee Country: Australia
Flowchart:
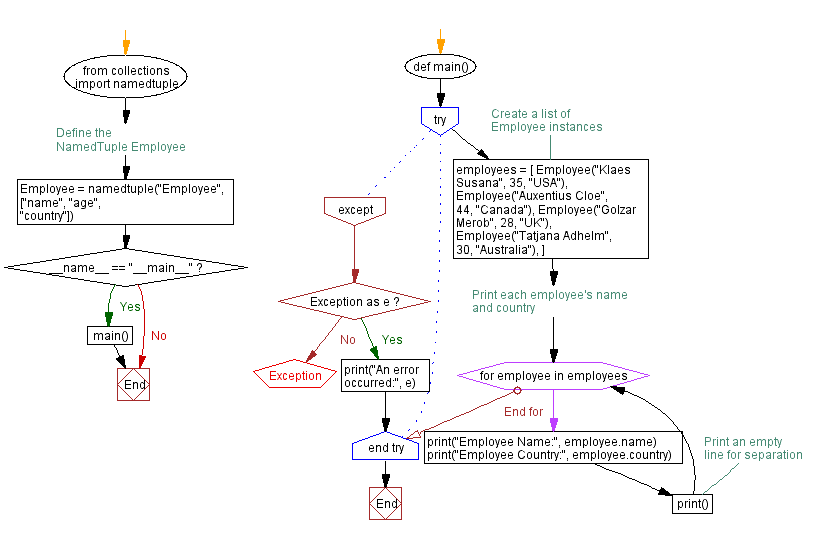
For more Practice: Solve these Related Problems:
- Write a Python program to define a NamedTuple `Employee` with fields: name, age, country, and department, then print each employee's name and country.
- Write a Python function that creates a list of `Employee` NamedTuples and returns a dictionary mapping each country to a list of employee names.
- Write a Python script to filter a list of `Employee` NamedTuples by a minimum age and print the names of employees from a specified country.
- Write a Python program to sort a list of `Employee` NamedTuples by age and then print each employee's name and country in sorted order.
Go to:
Previous: Python Extended Data Type NamedTuple Exercises Home.
Next: Python NamedTuple example: Point coordinates.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.