Python NamedTuple example: Point coordinates
2. Point NamedTuple
Write a Python program that defines a NamedTuple called Point with fields x, y and z representing the coordinate of a point. Access and print the fields.
Sample Solution:
Code:
from collections import namedtuple
# Define a NamedTuple named 'Point' with fields 'x', 'y', and 'z'
Point = namedtuple("Point", ["x", "y", "z"])
# Create an instance of the Point NamedTuple
p = Point(4, 7, 10)
# Access and print fields using dot notation
print("x:", p.x)
print("y:", p.y)
print("z:", p.z)
Output:
x: 4 y: 7 z: 10
In the exercise above, we first define a NamedTuple named 'Point' with fields x, y, and z. Then, we create an instance of the 'Point' NamedTuple with specific values for each field. Finally, we access and print the fields' values using dot notation.
Flowchart:
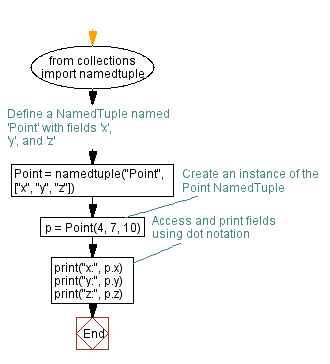
For more Practice: Solve these Related Problems:
- Write a Python program to define a NamedTuple `Point` with fields: x, y, and z, then compute the Euclidean distance from the point to the origin.
- Write a Python function that takes a list of `Point` NamedTuples and returns the point with the maximum x-coordinate.
- Write a Python script to define several `Point` NamedTuples and then calculate the midpoint between two given points.
- Write a Python program to sort a list of `Point` NamedTuples by their z-coordinate and print the sorted list.
Go to:
Previous: Python NamedTuple example: Employee information.
Next: Python NamedTuple example: Creating a food dictionary.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.