Python NamedTuple example: Creating a food dictionary
3. Food Dictionary with NamedTuple
Write a Python program that creates a dictionary where keys are food names and prices are instances of the Food named tuple.
Sample Solution:
Code:
from collections import namedtuple
# Define a NamedTuple named 'Food' with fields 'name' and 'price'
Food = namedtuple("Food", ["name", "price"])
# Create instances of the Food NamedTuple
food1 = Food("Pizza", 11.90)
food2 = Food("Burger", 7.45)
food3 = Food("Salad", 5.45)
# Create a dictionary where keys are food names and values are Food instances
food_dict = {
food1.name: food1,
food2.name: food2,
food3.name: food3
}
# Access and print values using dictionary keys
for food_name, food_info in food_dict.items():
print(f"Food: {food_name}, Price: ${food_info.price:.2f}")
Output:
Food: Pizza, Price: $11.90 Food: Burger, Price: $7.45 Food: Salad, Price: $5.45
In the exercise above, we first define a NamedTuple named "Food" with the fields 'name' and 'price'. We then create instances of the "Food" NamedTuple with different food items and their prices. Next, we create a dictionary named 'food_dict', whose keys are food names and whose values are instances of the "Food" NamedTuple. Finally, we iterate through the dictionary and print the food names and their corresponding prices.
Flowchart:
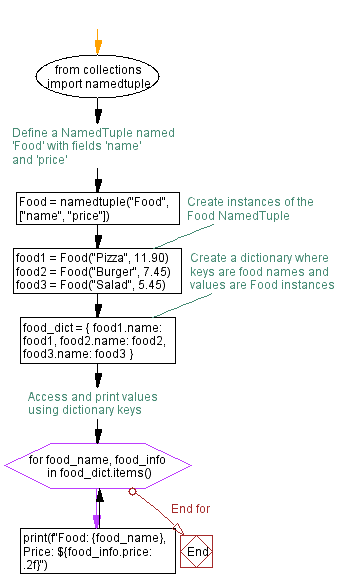
For more Practice: Solve these Related Problems:
- Write a Python program that defines a NamedTuple 'Food' with fields: name, price, and category, then creates a dictionary with food names as keys and 'Food' NamedTuples as values, and prints the dictionary.
- Write a Python script to filter a dictionary of 'Food' NamedTuples by a price range and output the names and prices of foods within that range.
- Write a Python program to update the price of a specific food in a dictionary where the values are 'Food' NamedTuples, then print the updated dictionary.
- Write a Python function to group 'Food' NamedTuples from a dictionary by category and print the resulting groups.
Previous: Python NamedTuple example: Point coordinates.
Next: Python NamedTuple example: Defining a student NamedTuple.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.