Python Removing key-Value pair from OrderedDict
Write a Python function that creates an OrderedDict and removes a key-value pair from the OrderedDict using a given key.
Sample OrderedDict:
'Laptop': 40
'Desktop': 45
'Mobile': 35
'Charger': 25
Sample Solution:
Code:
from collections import OrderedDict
def remove_key_from_ordered_dict(od, key_to_remove):
if key_to_remove in od:
del od[key_to_remove]
print(f"Key '{key_to_remove}' removed successfully.")
else:
print(f"Key '{key_to_remove}' not found in the OrderedDict.")
# Create an OrderedDict
ordered_dict = OrderedDict([
('Laptop', 40),
('Desktop', 45),
('Mobile', 35),
('Charger', 25)
])
print("Original OrderedDict: ",ordered_dict)
# Remove a key-value pair from the OrderedDict
print("\nKey to remove: 'Desktop'")
key_to_remove = 'Desktop'
remove_key_from_ordered_dict(ordered_dict, key_to_remove)
# Print the updated OrderedDict
print("\nUpdated OrderedDict: ",ordered_dict)
Output:
Original OrderedDict: OrderedDict([('Laptop', 40), ('Desktop', 45), ('Mobile', 35), ('Charger', 25)]) Key to remove: 'Desktop' Key 'Desktop' removed successfully. Updated OrderedDict: OrderedDict([('Laptop', 40), ('Mobile', 35), ('Charger', 25)])
In the exercise above, the "remove_key_from_ordered_dict()" function takes an 'OrderedDict' and a key to remove as inputs. If the key exists in the 'OrderedDict', the function deletes the corresponding key-value pair using the 'del' statement. It prints a message if the key does not exist. Finally the function prints the updated 'OrderedDict' after removing the key-value pair.
Flowchart:
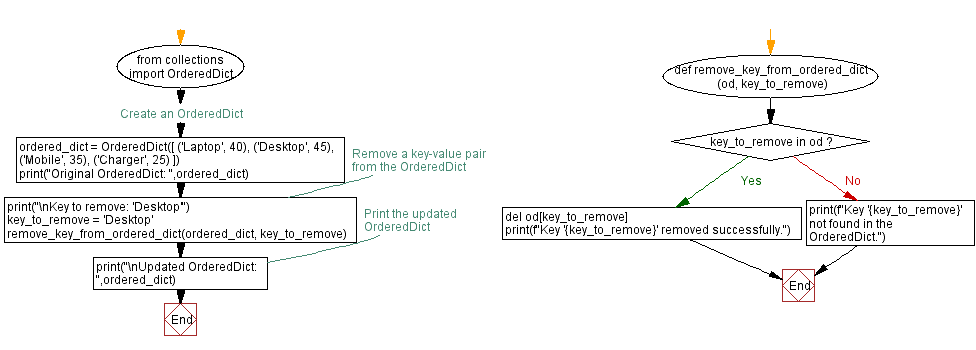
Previous: Python merging OrderedDicts with summed values.
Next: Python generating random ASCII OrderedDict.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics