Python function to handle empty strings
1. Return None for Empty String
Write a Python function that takes a string as input and returns "None" if the string is empty, otherwise it returns the given string.
Sample Solution:
Code:
def check_string(input_string):
if not input_string:
return "None"
return input_string
def main():
try:
input_string = input("Input a string: ")
result = check_string(input_string)
print("Result:", result)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Input a string: Result: None
Input a string: python Result: python
In the above exercise the program defines the "check_string()" function, which checks if the input string is empty. If it is, it returns the string "None", otherwise, it returns the original input string. The "main()" function takes user input, processes it using the "check_string()" function, and prints the result.
Flowchart:
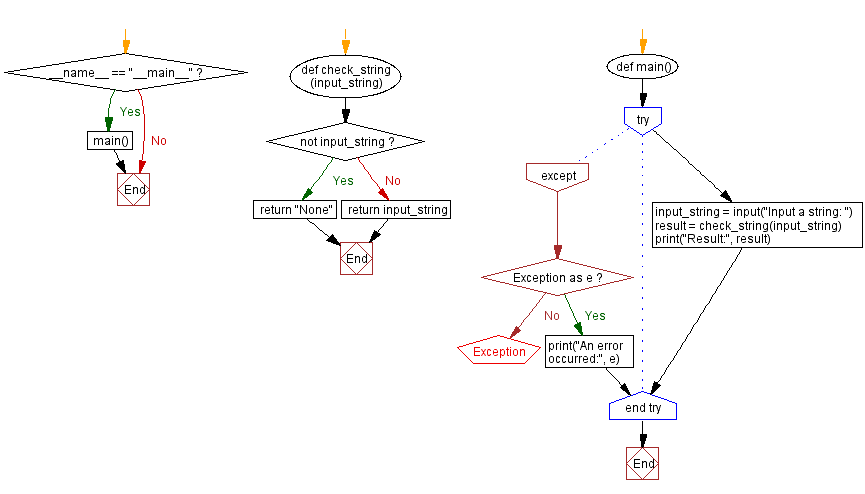
For more Practice: Solve these Related Problems:
- Write a Python function that accepts a string and returns "None" if the string is empty or consists only of whitespace, otherwise returns the string unchanged.
- Write a Python program to prompt the user for input and use boolean logic to return None if the input is empty; otherwise, print the input.
- Write a Python function that tests if a string is empty using its truth value and returns None, and then use it to filter a list of strings.
- Write a Python program that incorporates error checking by returning None for an empty string and a default message for strings that fail a length check.
Go to:
Previous: Python Extended Data Type None Exercises Home.
Next: Python function to retrieve middle character
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.