Python string substring replacement
10. Substring Replace with None Check
Write a Python function that replaces all occurrences of a substring in a string with another substring. Returns None if the original string is empty.
Sample Solution:
Code:
def replace_substr(original_string, old_substring, new_substring):
if original_string:
return original_string.replace(old_substring, new_substring)
else:
return None
def main():
try:
input_string = "Java Exercises!"
old_substr = "Java"
new_substr = "Python"
result = replace_substr(input_string, old_substr, new_substr)
if result is None:
print("Original string is empty.")
else:
print("Original String:", input_string)
print("New String:", result)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Original String: Java Exercises! New String: Python Exercises!
Original string is empty.
In the exercise above, the "replace_substr()" function checks if the original string is not blank. If it's not empty, it uses the 'replace' method to replace all occurrences of the 'old_substr' with the 'new_substr'. If the original string is empty, it returns 'None'. The "main()" function demonstrates the function's usage by providing a sample string and printing the initial and modified strings.
Flowchart:
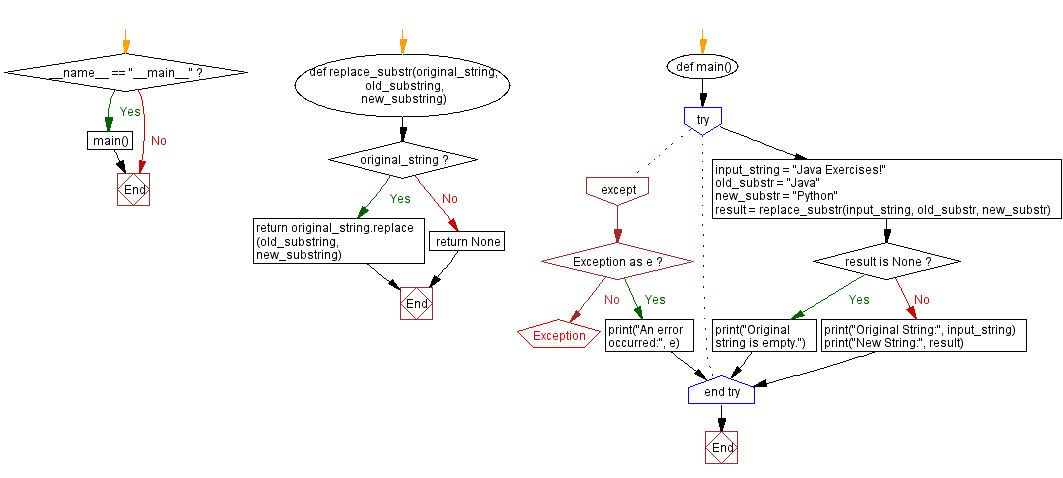
For more Practice: Solve these Related Problems:
- Write a Python program to replace all occurrences of a specified substring in a given string with another substring, returning None if the original string is empty.
- Write a Python function that checks if a string is empty before performing a substring replacement and returns None if it is.
- Write a Python script to implement a function that substitutes a target substring with a new substring in a string, handling empty strings by returning None.
- Write a Python program to replace multiple occurrences of a substring in a text and ensure that if the original text is empty, the function returns None.
Go to:
Previous:Insert None between list elements.
Next: Python Bytes and Byte arrays Home
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.