Python function to retrieve middle character
2. Middle Character or None
Write a Python function that returns the middle character of a string or "None" if the string length is odd.
Sample Solution:
Code:
def get_middle_character(input_string):
length = len(input_string)
if length % 2 == 0:
return "None"
else:
middle_index = length // 2
return input_string[middle_index]
def main():
try:
input_string = input("Input a string: ")
result = get_middle_character(input_string)
if result == "None":
print("String length is even, no middle character.")
else:
print("Middle character:", result)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Input a string: abcd String length is even, no middle character.
Input a string: abcde Middle character: c
In the above exercise, the program defines the "get_middle_character()" function, which calculates the length of the input string and checks if it's odd. If it's odd, it returns the middle character of the string; otherwise, it returns "None". In the "main()" function, user input is processed using "get_middle_character()", and the result is printed.
Flowchart:
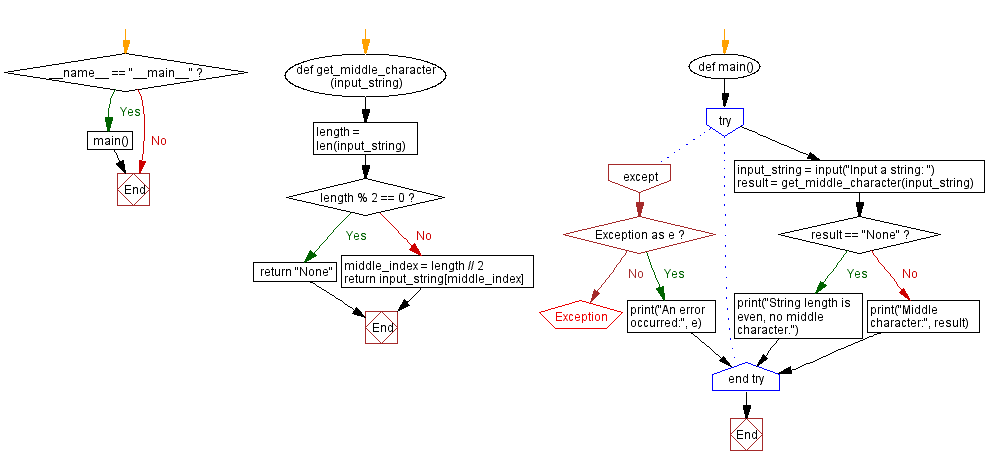
For more Practice: Solve these Related Problems:
- Write a Python function that returns the middle character of an even-length string, or None if the string length is odd.
- Write a Python program that extracts the middle character from a string only if the string length is even, and otherwise returns None.
- Write a Python function that handles edge cases by returning None for strings with odd lengths and prints a custom message for even-length strings.
- Write a Python script to take a list of strings and apply a function that returns the middle character if the string’s length is even, otherwise returns None.
Go to:
Previous: Python function to handle empty strings.
Next: Python program for handling empty strings.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.