Python dictionary value retrieval with key check
6. Dictionary Key Retrieval with Default
Write a Python program that defines a dictionary and retrieves a value using a key. If the key is not found, return None.
Sample Solution:
Code:
def retrieve_value(dictionary, key):
if key in dictionary:
return dictionary[key]
else:
return None
def main():
try:
person_data = {"name": "Bonifaas Shyam", "age": 25, "city": "Paris"}
#person_data = {"name": "Bonifaas Shyam", "city": "Paris"}
search_key = "age"
result = retrieve_value(person_data, search_key)
if result is None:
print(f"Key '{search_key}' not found.")
else:
print(f"The value for key '{search_key}' is:", result)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
The value for key 'age' is: 25
Key 'age' not found.
In the exercise above, the "retrieve_value()" function checks if the provided key exists in the dictionary. If it exists, it returns the corresponding value; otherwise, it returns None. The "main()" function demonstrates the function's usage by providing a sample dictionary and a search key. As soon as the key is found, or if it is not found, the value is printed.
Flowchart:
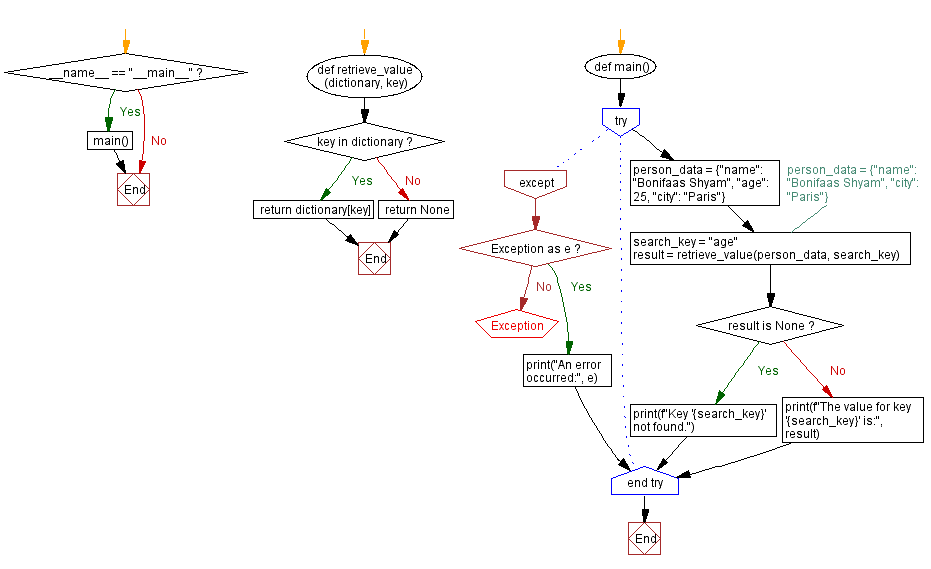
For more Practice: Solve these Related Problems:
- Write a Python program that defines a dictionary and retrieves a value for a specified key, returning None if the key does not exist.
- Write a Python function to safely access a dictionary value by key and return None when the key is missing, without raising an exception.
- Write a Python script to iterate over a list of keys, retrieving corresponding dictionary values and returning None for any missing keys.
- Write a Python program to use the dictionary.get() method to fetch values and default to None if the key is not found.
Go to:
Previous: Handle empty input.
Next: Count None values in list.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.