Python function: Insert None between list elements
Python None Data Type: Exercise-9 with Solution
Write a Python function that takes a list and returns a new list with None inserted between each element.
Sample Solution:
Code:
def insert_none(input_list):
new_list = []
for item in input_list:
new_list.append(item)
new_list.append(None)
new_list.pop() # Remove the last None added
return new_list
def main():
try:
original_list = [2, 4, 6, 8, 10]
new_list = insert_none(original_list)
print("Original List:", original_list)
print("New List with None:", new_list)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Original List: [2, 4, 6, 8, 10] New List with None: [2, None, 4, None, 6, None, 8, None, 10]
In the exercise above, the "insert_none()" function iterates through the input list and appends each element to the new list, followed by a 'None'. After iterating through all elements, it removes the last 'None' added. Using a sample list and printing the original and new lists, the "main()" function demonstrates the function's usage.
Flowchart:
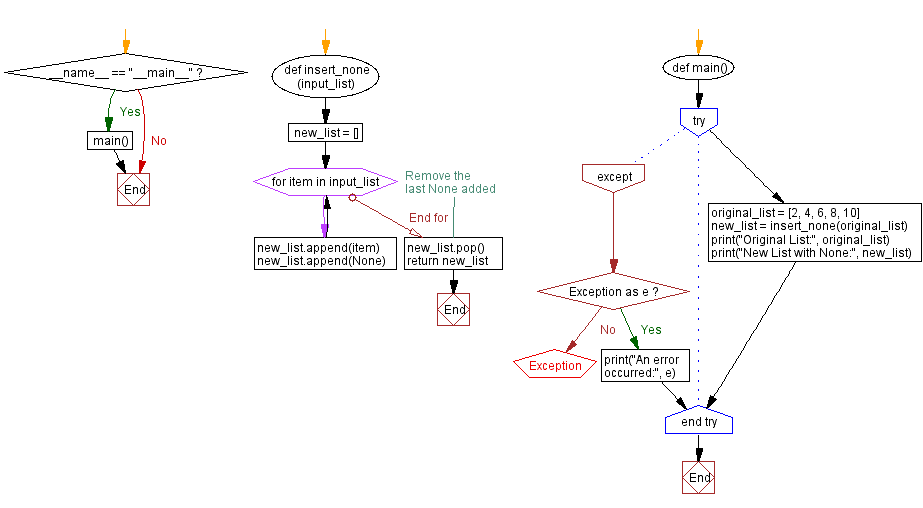
Previous: Sum with None check.
Next: Python string substring replacement.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/extended-data-types/python-extended-data-types-none-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics