Python function to filter even numbers
1. Even Number Filter
Write a Python function that filters out even numbers from a list of integers using the filter function.
Sample Solution:
Python Code:
def filter_even_nums(nums):
"""
Filters out even nums from a list of integers.
Args:
nums (list): A list of integers.
Returns:
list: A new list containing only the odd numbers.
"""
# Define the filtering function
def is_odd(num):
return num % 2 != 0
# Use the filter function to filter out even numbers
odd_nums = list(filter(is_odd, nums))
return odd_nums
nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print("Original numbers:",nums)
result = filter_even_nums(nums)
print("After filters out even numbers from the said list of integers ")
print(result) # Output: [1, 3, 5, 7, 9]
Explanation:
In the exercise above -
- The "filter_even_numbers()" function takes a list of integers called numbers as input.
- Inside the function, we define a nested function "is_odd()" that returns True for odd numbers (numbers not divisible by 2).
- Next use the filter function to filter out even numbers from the numbers list by applying the is_odd function as the filtering condition.
- The filtered odd numbers are converted to a list and returned as the result.
Sample Output:
Original numbers: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] After filters out even numbers from the said list of integers [1, 3, 5, 7, 9]
Flowchart:
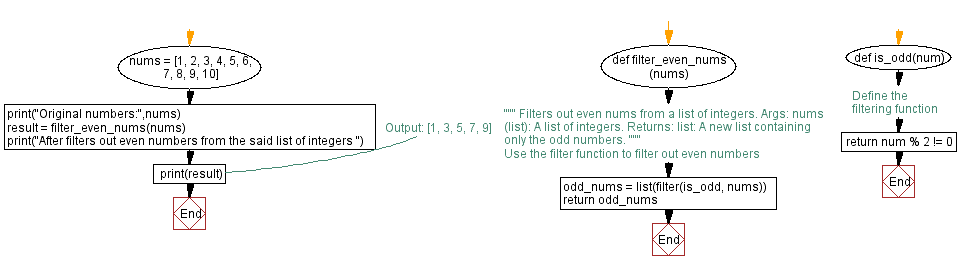
For more Practice: Solve these Related Problems:
- Write a Python program to filter out even numbers from a nested list of integers by first flattening the list and then applying the filter function.
- Write a Python function that filters out even numbers from a list only if they exceed a given threshold, using the filter function.
- Write a Python program that uses the filter function to remove even numbers from a list and then converts the remaining odd numbers to their string representations.
- Write a Python program to filter out even numbers from a list and then compute the sum of the odd numbers using the filter function.
Python Code Editor:
Previous: Python Filter Exercise Home
Next: Python program to extract uppercase letters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.