Python program to extract uppercase letters
Python Filter: Exercise-2 with Solution
Write a Python program that uses the filter function to extract all uppercase letters from a list of mixed-case strings.
Sample Solution:
Python Code:
# Define a list of mixed-case strings
mixed_case_strings = ["Hello", "w3resource", "Python", "Filter", "Learning"]
print("Original list of strings:\n",mixed_case_strings)
# Use the filter function to extract uppercase letters
uppercase_letters = list(filter(lambda char: char.isupper(), ''.join(mixed_case_strings)))
print("\nExtract all uppercase letters from the said list of mixed-case strings:")
# Print the extracted uppercase letters
print(uppercase_letters)
Explanation:
In the exercise above,
- First, start with a list of mixed-case strings named mixed_case_strings.
- Next, we use the filter function to filter characters from all strings and retain only uppercase letters. We do this by using a lambda function that checks if a character is uppercase using the isupper() method.
- The filter function returns an iterable of uppercase letters, which we convert to a list.
- Finally, we print the extracted uppercase letters.
Sample Output:
Original list of strings: ['Hello', 'w3resource', 'Python', 'Filter', 'Learning'] Extract all uppercase letters from the said list of mixed-case strings: ['H', 'P', 'F', 'L']
Flowchart:
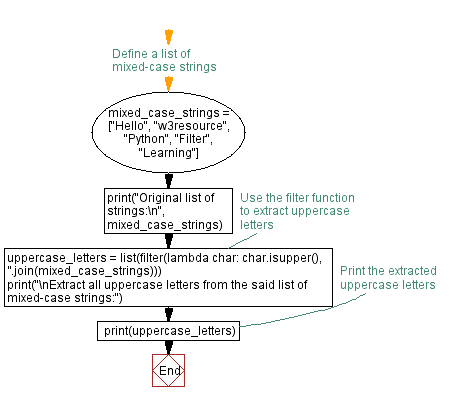
Python Code Editor:
Previous: Python function to filter even numbers.
Next:Python function to filter numbers by threshold.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/filter/python-filter-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics